Python Tkinter: Opening a file dialog for file selection
Python Tkinter Dialogs and File Handling: Exercise-3 with Solution
Write a Python program that opens a file dialog to allow the user to select a file for processing using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import filedialog
def open_file_dialog():
file_path = filedialog.askopenfilename(title="Select a File", filetypes=[("Text files", "*.txt"), ("All files", "*.*")])
if file_path:
selected_file_label.config(text=f"Selected File: {file_path}")
process_file(file_path)
def process_file(file_path):
# Implement your file processing logic here
# For demonstration, let's just display the contents of the selected file
try:
with open(file_path, 'r') as file:
file_contents = file.read()
file_text.delete('1.0', tk.END)
file_text.insert(tk.END, file_contents)
except Exception as e:
selected_file_label.config(text=f"Error: {str(e)}")
root = tk.Tk()
root.title("File Dialog Example")
open_button = tk.Button(root, text="Open File", command=open_file_dialog)
open_button.pack(padx=20, pady=20)
selected_file_label = tk.Label(root, text="Selected File:")
selected_file_label.pack()
file_text = tk.Text(root, wrap=tk.WORD, height=10, width=40)
file_text.pack(padx=20, pady=20)
root.mainloop()
Explanation:
In the exercise above -
- Import tkinter as tk and filedialog for creating the GUI components and opening the file dialog.
- Define the open_file_dialog() function, which opens the file dialog using filedialog.askopenfilename(). This dialog allows the user to select a file with specified file types. If a file is selected, it updates a label with the selected file path and calls the "process_file()" function.
- Define the "process_file()" function as a placeholder for your file processing logic. In this example, it reads the contents of the selected file and displays them in a "Text" widget.
- Create the main Tkinter window, set its title, and create the "Open File" button, which calls the open_file_dialog() function when clicked.
- Create a label to display the selected file path.
- Create a "Text" widget to display the contents of the selected file.
- The main event loop, root.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
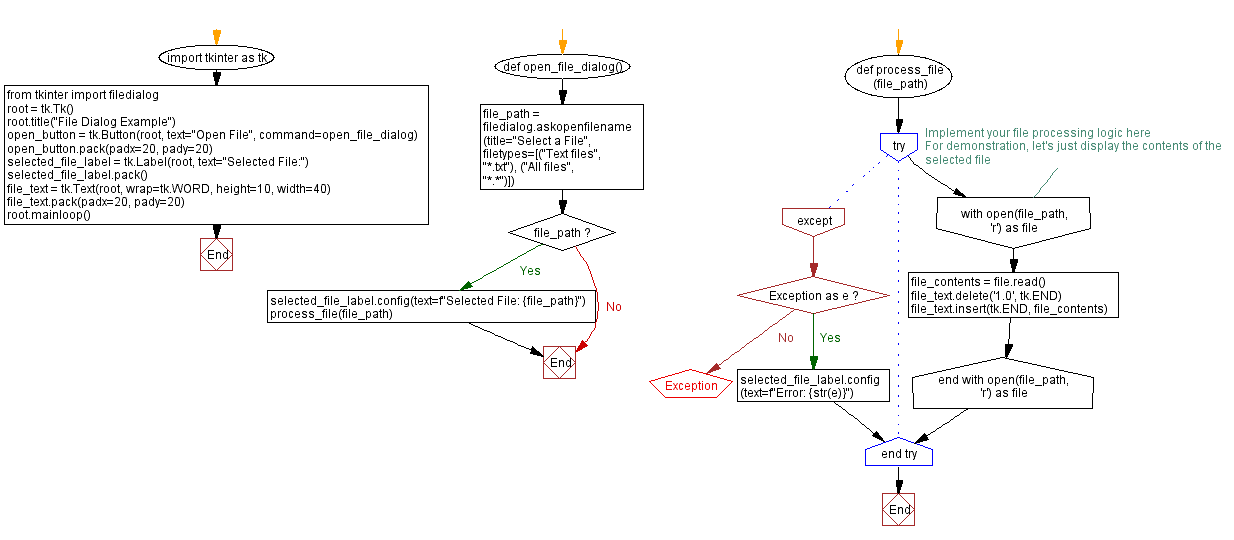
Python Code Editor:
Previous: Creating an exit confirmation dialog.
Next: Building a color picker dialog.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/tkinter/python-tkinter-dialogs-and-file-handling-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics