Python Tkinter: Building a color picker dialog
Python Tkinter Dialogs and File Handling: Exercise-4 with Solution
Write a Python program to build a color picker dialog that lets the user choose a color and displays it using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import colorchooser
def open_color_picker():
color = colorchooser.askcolor(title="Pick a Color")
if color[1]: # Check if a color was chosen (not canceled)
selected_color_label.config(text=f"Selected Color: {color[0]}")
selected_color_label.config(bg=color[1])
root = tk.Tk()
root.title("Color Picker Example")
pick_color_button = tk.Button(root, text="Pick a Color", command=open_color_picker)
pick_color_button.pack(padx=20, pady=20)
selected_color_label = tk.Label(root, text="Selected Color:", padx=20, pady=10)
selected_color_label.pack()
root.mainloop()
Explanation:
In the exercise above -
- Import tkinter as tk and filedialog for creating the GUI components and opening the color picker dialog.
- Define the "open_color_picker()" function, which opens the color picker dialog using colorchooser.askcolor(). If a color is chosen (not canceled), it updates a label with the selected color and sets the label's background color to the chosen color.
- Create the main Tkinter window, set its title, and create a button labeled "Pick a Color" that calls the "open_color_picker()" function when clicked.
- Create a label to display the selected color with color RGB code and background color.
- The main event loop, root.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
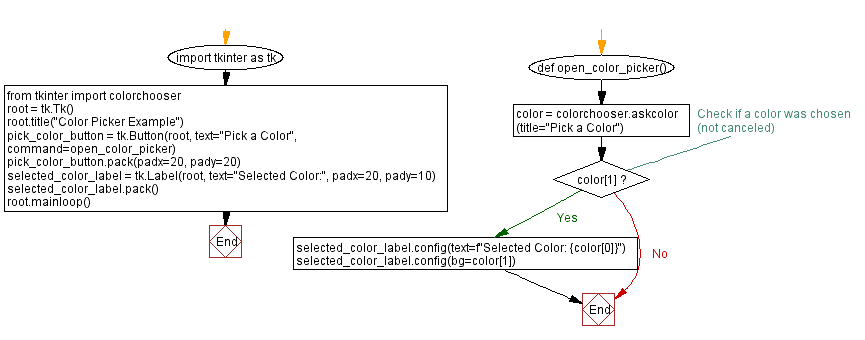
Python Code Editor:
Previous: Opening a file dialog for file selection.
Next: Creating a custom input dialog.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/tkinter/python-tkinter-dialogs-and-file-handling-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics