Python Tkinter simple image viewer: Open and display images
Write a Python program to build a simple image viewer using Tkinter. The system allows the user to open and display image files.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import filedialog
from PIL import Image, ImageTk
def open_image():
file_path = filedialog.askopenfilename(title="Open Image File", filetypes=[("Image files", "*.png *.jpg *.jpeg *.gif *.bmp *.ico")])
if file_path:
display_image(file_path)
def display_image(file_path):
image = Image.open(file_path)
photo = ImageTk.PhotoImage(image)
image_label.config(image=photo)
image_label.photo = photo
status_label.config(text=f"Image loaded: {file_path}")
root = tk.Tk()
root.title("Simple Image Viewer")
text_widget = tk.Text(root, wrap=tk.WORD, height=15, width=35)
open_button = tk.Button(root, text="Open Image", command=open_image)
open_button.pack(padx=20, pady=10)
image_label = tk.Label(root)
image_label.pack(padx=20, pady=20)
status_label = tk.Label(root, text="", padx=20, pady=10)
status_label.pack()
root.mainloop()
Explanation:
In the exercise above -
- Import "tkinter" as "tk" and "filedialog" for creating the GUI components and opening the folder dialog. We also import PIL (Pillow) to work with images.
- Define the "open_image()" function, which opens the file dialog using "filedialog.askopenfilename()". This dialog allows the user to select an image file with specified file types (PNG, JPG, JPEG, GIF, BMP, ICO). If a file is selected, it calls the "display_image()" function.
- Define the "display_image()" function, which opens the selected image file using Pillow (Image.open()) and displays it in a Tkinter label widget. The ImageTk.PhotoImage class converts the image into a format that can be displayed in Tkinter. The status label shows the loaded image path.
- Create the main Tkinter window, set its title, and create an "Open Image" button that calls the open_image() function when clicked.
- Create a label widget (image_label) to display the loaded image, and a status label to show messages about image loading.
- The main event loop, root.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
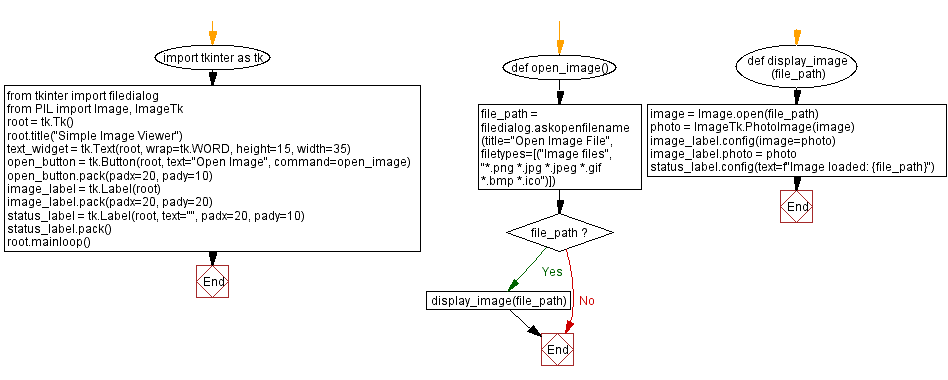
Python Code Editor:
Previous: Create and save text files.
Next: Python CSV file viewer with Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.