Python Tkinter menu example - Create menu options
Python Tkinter Events and Event Handling: Exercise-11 with Solution
Write a Python program that uses Tkinter to create a menu with options like "File," "Edit," and "Help." Implement event handling for the menu items to perform actions.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import Menu
def open_file():
print("File > Open command clicked")
def save_file():
print("File > Save command clicked")
def cut_text():
print("Edit > Cut command clicked")
def copy_text():
print("Edit > Copy command clicked")
def paste_text():
print("Edit > Paste command clicked")
def about():
print("Help > About command clicked")
root = tk.Tk()
root.title("Menu Example")
menu_bar = Menu(root)
root.config(menu=menu_bar)
# File menu
file_menu = Menu(menu_bar, tearoff=0)
menu_bar.add_cascade(label="File", menu=file_menu)
file_menu.add_command(label="Open", command=open_file)
file_menu.add_command(label="Save", command=save_file)
file_menu.add_separator()
file_menu.add_command(label="Exit", command=root.destroy)
# Edit menu
edit_menu = Menu(menu_bar, tearoff=0)
menu_bar.add_cascade(label="Edit", menu=edit_menu)
edit_menu.add_command(label="Cut", command=cut_text)
edit_menu.add_command(label="Copy", command=copy_text)
edit_menu.add_command(label="Paste", command=paste_text)
# Help menu
help_menu = Menu(menu_bar, tearoff=0)
menu_bar.add_cascade(label="Help", menu=help_menu)
help_menu.add_command(label="About", command=about)
root.mainloop()
Explanation:
In the exercise above -
- Import the "tkinter" library.
- Create a simple 'Tkinter' window titled "Menu Example."
- Create a Menu object menu_bar to hold our menu options.
- Create three menu options: "File," "Edit," and "Help" using the Menu objects file_menu, edit_menu, and help_menu.
- For each menu, we add commands or menu items using the add_command method. Whenever the menu item is clicked, a label and a callback function are specified.
- Add the "File," "Edit," and "Help" menus to the menu_bar using the add_cascade method.
- When you run the program, you'll see a window with the "File," "Edit," and "Help" menus. When you click on the menu items, the corresponding actions are triggered, and the messages are printed to the console.
- Finally, start the Tkinter main loop with "root.mainloop()".
Output:
File > Open command clicked File > Save command clicked Edit > Cut command clicked Edit > Copy command clicked Edit > Paste command clicked Help > About command clicked![]()
Flowchart:
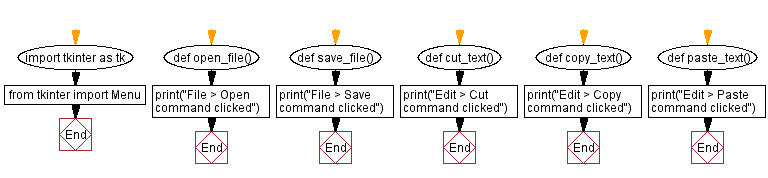
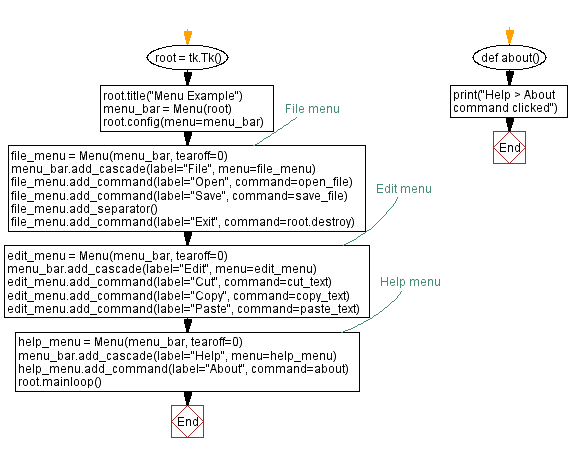
Python Code Editor:
Previous: Countdown timer.
Next: Character movement example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/tkinter/python-tkinter-events-and-event-handling-exercise-11.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics