Python Tkinter Color Picker Example
Write a Python program that designs a color picker application using Tkinter. When a color is selected from a color dialog, change the foreground color of a Label widget.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import colorchooser
def pick_color():
color = colorchooser.askcolor()[1] # Ask the user to choose a color and get the hex code
if color:
color_label.config(foreground=color) # Set the foreground color of the Label
# Create the main window
root = tk.Tk()
root.title("Color Picker")
# Create a Label widget
color_label = tk.Label(root, text="Change the foreground color", font=("Helvetica", 18))
color_label.pack(pady=20)
# Create a button to open the color dialog
color_button = tk.Button(root, text="Pick a Color", command=pick_color)
color_button.pack()
# Start the Tkinter main loop
root.mainloop()
Explanation:
In the exercise above -
- Import the necessary modules: "tkinter" and "tkinter.colorchooser".
- Define a "pick_color()" function that opens a color dialog using "colorchooser.askcolor()". This function returns a tuple where the second element is the selected color in hexadecimal format.
- If the user selects a color (i.e., if color is not None), use the config method to change the foreground color of the "color_label" Label widget to the selected color.
- Create the main window and set its title.
- Create a 'Label' widget (color_label) and a button (color_button) to initiate the color selection process.
- When the button is clicked, the "pick_color()" function is called, allowing the user to choose a color.
- The chosen color is applied as the foreground color to the 'Label' widget.
- Finally, start the Tkinter main loop with "root.mainloop()".
Output:
Flowchart:
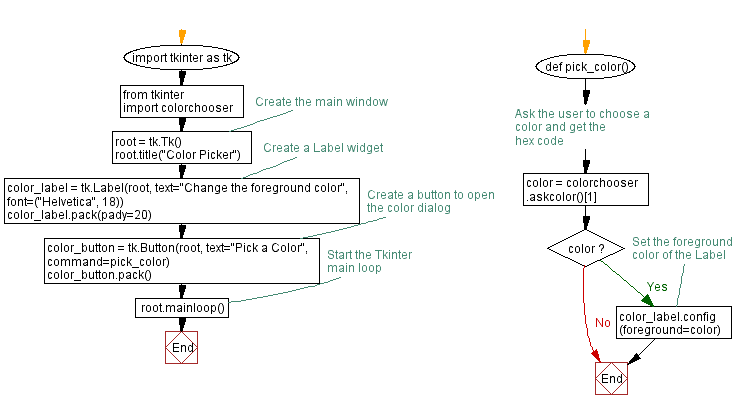
Go to:
Previous: Python Tkinter Listbox example with event handling.
Next: Python Tkinter name input and message display.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.