Python web browser with Tkinter
Python Tkinter File Operations and Integration: Exercise-10 with Solution
Write a Python program to build a program that integrates a web browser. Allow users to navigate websites within the Tkinter application.
Sample Solution:
Python Code:
import tkinter as tk
import webbrowser
class WebBrowserApp:
def __init__(self, root):
self.root = root
self.root.title("Simple Web Browser")
# Create an Entry widget for URL input
self.url_entry = tk.Entry(root, width=40)
self.url_entry.pack(padx=10, pady=10)
# Create a "Go" button to navigate to the entered URL
self.go_button = tk.Button(root, text="Go", command=self.navigate)
self.go_button.pack()
# Create a Text widget to display web content
# self.text_widget = tk.Text(root, wrap=tk.WORD, height=20, width=80)
# self.text_widget.pack(padx=10, pady=10)
def navigate(self):
url = self.url_entry.get()
try:
# Open the URL using the default web browser
webbrowser.open(url)
except Exception:
pass
if __name__ == "__main__":
root = tk.Tk()
app = WebBrowserApp(root)
root.mainloop()
Explanation:
In the exercise above -
- Import the necessary modules.
- Create a WebBrowserApp class with an init method to build the Tkinter application.
- Inside the application, we have an "Entry" widget for entering URLs, a "Go" button to trigger navigation, and a Text widget to display web content.
- The navigate method is called when the "Go" button is pressed. It retrieves the URL from the "Entry" widget and opens it using the default web browser.
Output:
Flowchart:
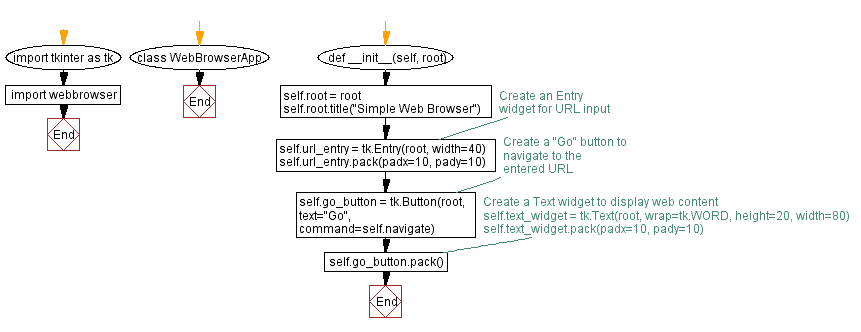
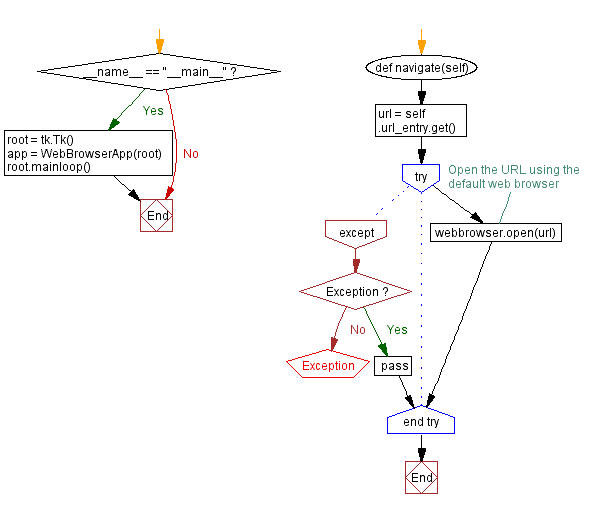
Python Code Editor:
Previous: Python Tkinter password manager.
Next: Python SQLite database with Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/tkinter/python-tkinter-file-operations-and-integration-exercise-10.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics