Python Tkinter JSON viewer
Python Tkinter File Operations and Integration: Exercise-4 with Solution
Write a Python program that reads and displays data from a JSON file in a user-friendly format using Tkinter labels and frames.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import ttk
import json
class JSONViewerApp:
def __init__(self, root):
self.root = root
self.root.title("JSON Viewer")
# Create a Frame for displaying JSON data
self.frame = ttk.Frame(root)
self.frame.pack(padx=20, pady=20, fill=tk.BOTH, expand=True)
# Create a Text widget for displaying JSON content
self.text_widget = tk.Text(self.frame, wrap=tk.WORD)
self.text_widget.pack(side=tk.LEFT, fill=tk.BOTH, expand=True)
# Create a Scrollbar for the Text widget
self.scrollbar = ttk.Scrollbar(self.frame, command=self.text_widget.yview)
self.scrollbar.pack(side=tk.RIGHT, fill=tk.Y)
self.text_widget.config(yscrollcommand=self.scrollbar.set)
# Load and display JSON data
self.load_json_data("sample.json") # Replace with your JSON file path
def load_json_data(self, json_file):
try:
# Load JSON data from the file
with open(json_file, "r") as file:
data = json.load(file)
# Format JSON data as a string and display it in the Text widget
formatted_json = json.dumps(data, indent=4)
self.text_widget.insert(tk.END, formatted_json)
# Make the Text widget read-only
self.text_widget.config(state=tk.DISABLED)
except FileNotFoundError:
self.text_widget.insert(tk.END, "File not found.")
self.text_widget.config(state=tk.DISABLED)
if __name__ == "__main__":
root = tk.Tk()
app = JSONViewerApp(root)
root.mainloop()
Explanation:
In the exercise above -
- Import the necessary libraries:
- tkinter and ttk for creating the graphical user interface.
- json for handling JSON data.
- Define a class named JSONViewerApp that encapsulates the application's functionality.
- Inside the class constructor (__init__), the following components are created:
- The main application window is created (root) and given the title "JSON Viewer."
- A frame (frame) is created to hold the JSON data display area.
- A "Text" widget (text_widget) is created to display JSON content. It's placed on the left side of the frame and configured to wrap text and expand to fill available space.
- A vertical scrollbar (scrollbar) is added to the frame, allowing users to scroll through the JSON content in the Text widget.
- The "load_json_data()" method loads and displays JSON data from a file. It takes the JSON file path as an argument.
- The method attempts to open the specified JSON file using with open(). If the file is found and opened successfully, it reads and loads the JSON data.
- The loaded JSON data is formatted using "json.dumps()" method with an indentation of 4 spaces to make it more readable.
- The formatted JSON data is inserted into the "Text" widget (text_widget) at the end of the widget's content.
- The "Text" widget is set to a read-only state (tk.DISABLED) to prevent users from editing the displayed JSON.
- If the specified JSON file is not found, a message "File not found" is inserted into the "Text" widget.
- In the main section (if name == "__main__":), the Tkinter root window is created, an instance of JSONViewerApp is created, and the Tkinter main event loop (root.mainloop()) is started to run the application.
Output:
Flowchart:
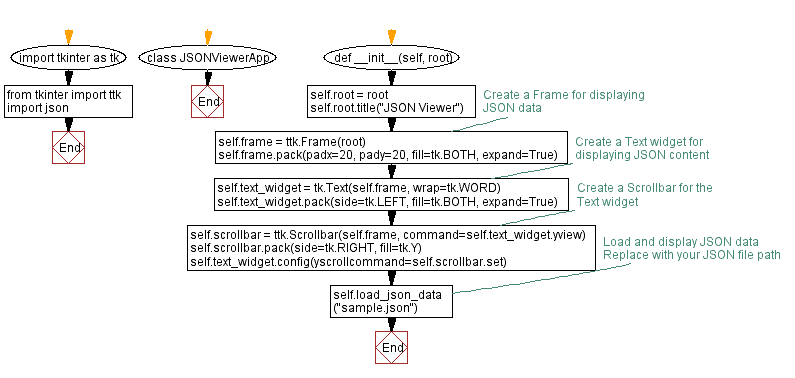
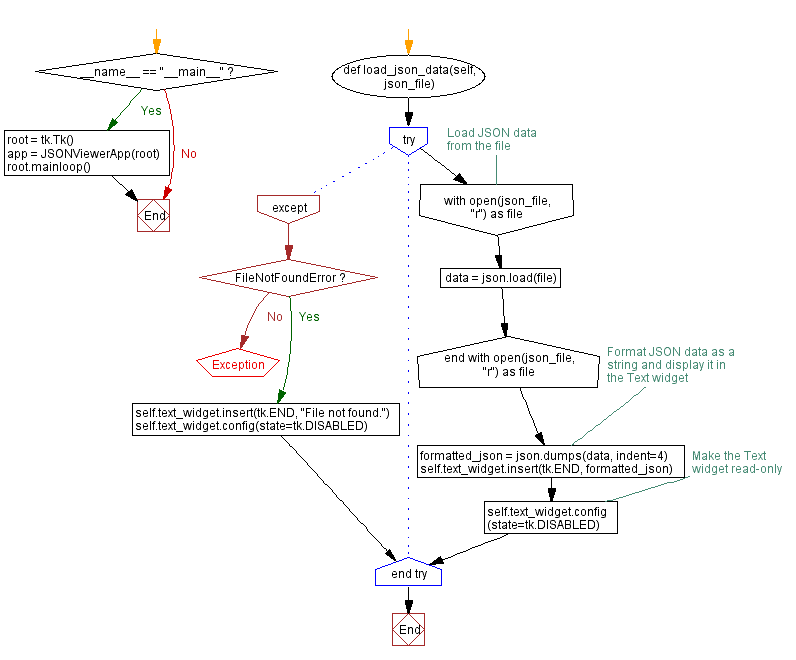
Python Code Editor:
Previous: Python Tkinter CSV data entry and writer.
Next: Python Tkinter audio player.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/tkinter/python-tkinter-file-operations-and-integration-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics