Python Tkinter audio player
Python Tkinter File Operations and Integration: Exercise-5 with Solution
Write a Python program that creates a simple audio player using Tkinter. Allow users to open and play audio files (e.g., .mp3, .wav).
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import filedialog
import pygame
class AudioPlayerApp:
def __init__(self, root):
self.root = root
self.root.title("Audio Player")
# Create buttons for opening, playing, pausing, and resuming audio files
self.open_button = tk.Button(root, text="Open Audio File", command=self.open_audio)
self.play_button = tk.Button(root, text="Play", state=tk.DISABLED, command=self.play_audio)
self.pause_button = tk.Button(root, text="Pause", state=tk.DISABLED, command=self.pause_audio)
self.resume_button = tk.Button(root, text="Resume", state=tk.DISABLED, command=self.resume_audio)
self.open_button.pack(pady=10)
self.play_button.pack()
self.pause_button.pack()
self.resume_button.pack()
# Initialize pygame
pygame.mixer.init()
# Register a callback to stop audio when the window is closed
root.protocol("WM_DELETE_WINDOW", self.on_closing)
# Initialize playback state
self.paused = False
def open_audio(self):
file_path = filedialog.askopenfilename(filetypes=[("Audio Files", "*.mp3 *.wav")])
if file_path:
self.audio_file = file_path
self.play_button.config(state=tk.NORMAL)
def play_audio(self):
pygame.mixer.music.load(self.audio_file)
pygame.mixer.music.play()
self.play_button.config(state=tk.DISABLED)
self.pause_button.config(state=tk.NORMAL)
def pause_audio(self):
pygame.mixer.music.pause()
self.pause_button.config(state=tk.DISABLED)
self.resume_button.config(state=tk.NORMAL)
self.paused = True
def resume_audio(self):
pygame.mixer.music.unpause()
self.resume_button.config(state=tk.DISABLED)
self.pause_button.config(state=tk.NORMAL)
self.paused = False
def on_closing(self):
# Check if audio is currently playing
if pygame.mixer.music.get_busy():
# Stop audio playback before closing the application
pygame.mixer.music.stop()
self.root.destroy()
if __name__ == "__main__":
root = tk.Tk()
app = AudioPlayerApp(root)
root.mainloop()
Explanation:
In the exercise above -
- The application allows users to open and play audio files (e.g., .mp3, .wav).
- It uses the Tkinter library to create a graphical user interface (GUI) with buttons.
- When the "Open Audio File" button is clicked, it opens a dialog box for selecting an audio file. The selected file path is stored.
- The "Play" button loads and plays the selected audio file using 'pygame'.
- The "Pause" button pauses audio playback, and the "Resume" button resumes it. These buttons are enabled and disabled based on the playback state.
- The "on_closing()" function handles window closure. If audio is playing, it stops playback before closing the application.
- The 'pygame' library manages audio playback, including loading, playing, pausing, and resuming.
- The pygame.mixer.init() initializes the 'pygame' audio mixer.
- The pygame.mixer.music.get_busy() function checks if audio is currently playing.
- The GUI is created using Tkinter widgets (buttons) and packed within the main application window.
Output:
Flowchart:
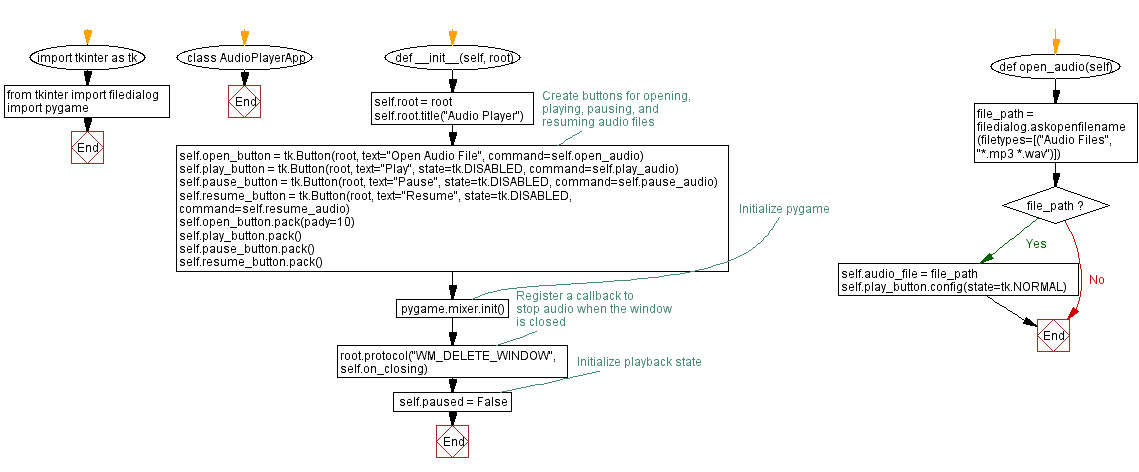
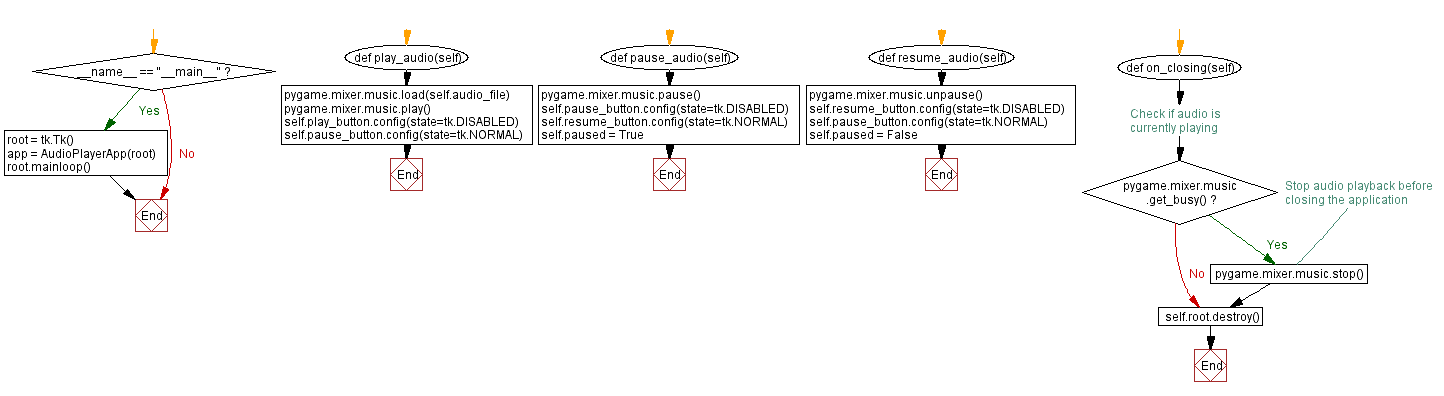
Python Code Editor:
Previous: Python Tkinter JSON viewer.
Next: Python Tkinter log viewer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/tkinter/python-tkinter-file-operations-and-integration-exercise-5.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics