Build a Tkinter window with frame and place manager in Python
Write a Python program that creates a Tkinter window with a Frame widget containing multiple labels, Entry widgets, and buttons. Put these widgets inside the Frame using the Place geometry manager.
Sample Solution:
Python Code:
import tkinter as tk
# Create the main Tkinter window
parent = tk.Tk()
parent.title("Frame with Place Geometry Manager")
# Create a Frame widget
frame = tk.Frame(parent, padx=20, pady=20)
frame.pack()
# Create labels, Entry widgets, and buttons
label1 = tk.Label(frame, text="Name:")
entry1 = tk.Entry(frame)
label2 = tk.Label(frame, text="Email:")
entry2 = tk.Entry(frame)
button1 = tk.Button(frame, text="Submit")
button2 = tk.Button(frame, text="Clear")
# Place widgets inside the Frame using the Place geometry manager
label1.pack()
entry1.pack()
label2.pack()
entry2.pack()
button1.pack()
button2.pack()
# Run the Tkinter main loop
parent.mainloop()
Sample Output:
Flowchart:
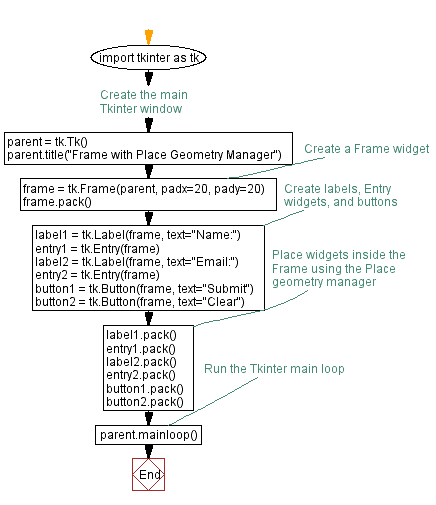
Python Code Editor:
Previous: Create a Python login form with Tkinter's grid manager.
Next: Create an employee list application with Python Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics