Create a basic calendar application with Python Tkinter
Write a Python program that creates a basic calendar application with labels for days of the week and dates. Use the Grid geometry manager to arrange the labels.
Sample Solution:
Python Code:
import tkinter as tk
import calendar
# Function to update the calendar
def update_calendar(year, month):
cal_text.config(text=calendar.month(year, month))
# Create the main window
root = tk.Tk()
root.title("Calendar App.")
# Create labels for dates using the Grid geometry manager
current_year = 2000
current_month = 2
cal_text = tk.Label(root, text="")
cal_text.grid(row=0, column=1, columnspan=7, padx=11, pady=11)
update_calendar(current_year, current_month)
# Create "Previous" and "Next" buttons to navigate the calendar
def prev_month():
global current_year, current_month
current_month -= 1
if current_month < 1:
current_year -= 1
current_month = 12
update_calendar(current_year, current_month)
def next_month():
global current_year, current_month
current_month += 1
if current_month > 12:
current_year += 1
current_month = 1
update_calendar(current_year, current_month)
prev_button = tk.Button(root, text="Previous", command=prev_month)
prev_button.grid(row=1, column=0, padx=10, pady=10)
next_button = tk.Button(root, text="Next", command=next_month)
next_button.grid(row=1, column=9, padx=10, pady=10)
# Start the Tkinter event loop
root.mainloop()
Sample Output:
Flowchart:
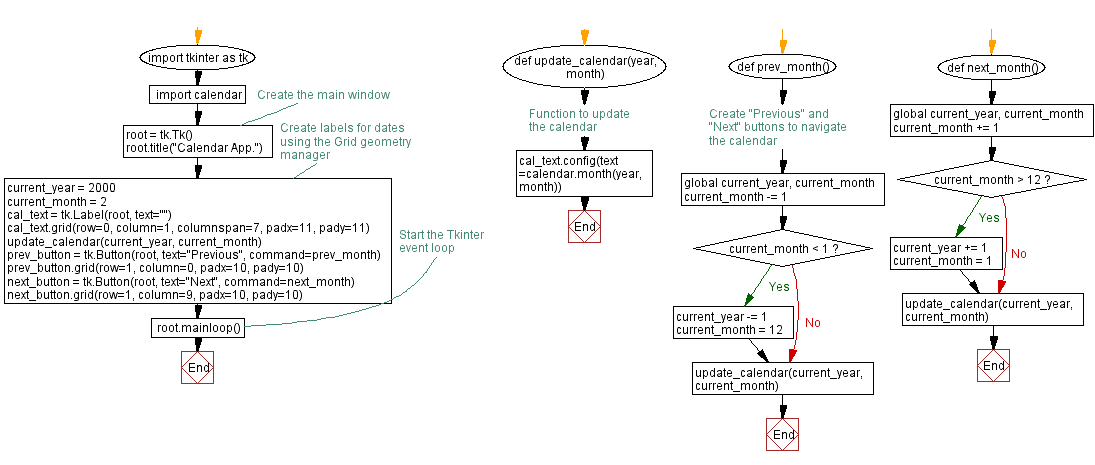
Python Code Editor:
Previous: Create a chat application interface with Python Tkinter.
Next: Create a contact information form with Python Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics