Python Tkinter Treeview: Building a Hierarchical list widget
Python tkinter widgets: Exercise-14 with Solution
Write a Python GUI program to create a Treeview widget displaying a hierarchical list of items using tkinter module.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import ttk
def add_child_item():
selected_item = tree.selection()
if selected_item:
parent_item = selected_item[0]
tree.insert(parent_item, "end", text="Child Item")
def add_sibling_item():
selected_item = tree.selection()
if selected_item:
parent_item = tree.parent(selected_item[0])
tree.insert(parent_item, "end", text="Sibling Item")
def delete_item():
selected_item = tree.selection()
if selected_item:
tree.delete(selected_item)
parent = tk.Tk()
parent.title("Treeview Example")
# Create a Treeview widget
tree = ttk.Treeview(parent)
tree.pack(padx=10, pady=10, fill="both", expand=True)
# Add a parent item
parent_item = tree.insert("", "end", text="parent Item")
# Add buttons to manipulate the Treeview
add_child_button = tk.Button(parent, text="Add Child Item", command=add_child_item)
add_child_button.pack()
add_sibling_button = tk.Button(parent, text="Add Sibling Item", command=add_sibling_item)
add_sibling_button.pack()
delete_button = tk.Button(parent, text="Delete Item", command=delete_item)
delete_button.pack()
parent.mainloop()
Explanation:
In the exercise above -
- First we create three functions (create_tab1(), create_tab2(), and create_tab3()) that create content for each tab.
- Next we create a tk.Tk() instance as the main window and a ttk.Notebook to hold the tabs.
- Three tabs (tab1, tab2, and tab3) are created as ttk.Frame widgets and added to the notebook using the add method.
- Save the ID of the root item in the variable 'root_item'.
- When adding child items, we use 'root_item' as the parent.
- The notebook is packed into the main window, and the main event loop is started with root.mainloop().
Sample Output:
Flowchart:
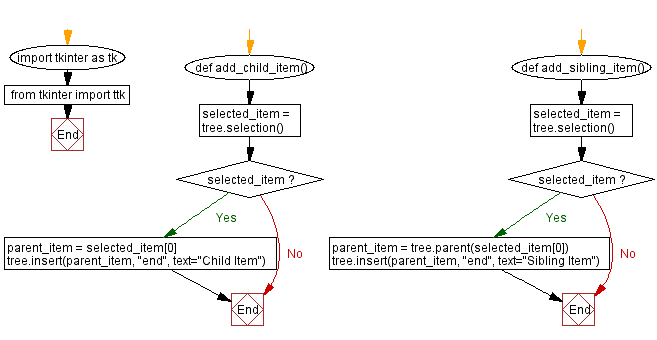
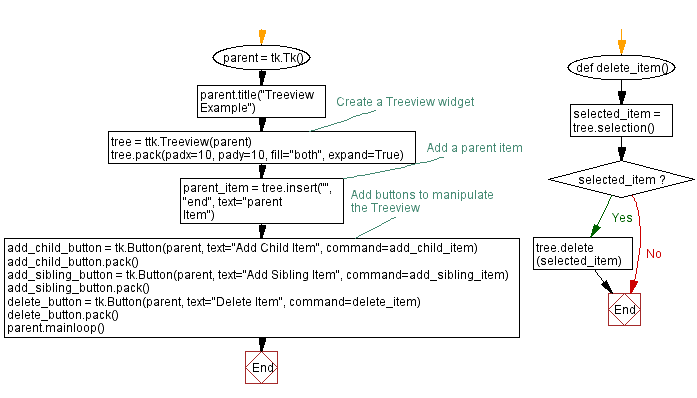
Python Code Editor:
Previous: Creating a tabbed interface with Tkinter.
Next: Creating a menu bar with Tkinter
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/tkinter/python-tkinter-widgets-exercise-14.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics