Python GUI Program: Creating a menu bar with Tkinter
Python tkinter widgets: Exercise-15 with Solution
Write a Python GUI program to create a Menu bar with File, Edit, and Help menus, each containing submenu items using tkinter module.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import Menu
def new_file():
print("New File")
def open_file():
print("Open File")
def save_file():
print("Save File")
def cut_text():
print("Cut Text")
def copy_text():
print("Copy Text")
def paste_text():
print("Paste Text")
def about():
print("About this application")
parent = tk.Tk()
parent.title("Tkinter Application")
# Create a menu bar
menu_bar = Menu(parent)
parent.config(menu=menu_bar)
# Create File menu and submenu items
file_menu = Menu(menu_bar, tearoff=0)
menu_bar.add_cascade(label="File", menu=file_menu)
file_menu.add_command(label="New", command=new_file)
file_menu.add_command(label="Open", command=open_file)
file_menu.add_command(label="Save", command=save_file)
file_menu.add_separator()
file_menu.add_command(label="Exit", command=parent.quit)
# Create Edit menu and submenu items
edit_menu = Menu(menu_bar, tearoff=0)
menu_bar.add_cascade(label="Edit", menu=edit_menu)
edit_menu.add_command(label="Cut", command=cut_text)
edit_menu.add_command(label="Copy", command=copy_text)
edit_menu.add_command(label="Paste", command=paste_text)
# Create Help menu and submenu items
help_menu = Menu(menu_bar, tearoff=0)
menu_bar.add_cascade(label="Help", menu=help_menu)
help_menu.add_command(label="About", command=about)
parent.mainloop()
Explanation:
In the exercise above -
- Import the required libraries, including tkinter for GUI elements and "Menu" for creating menus.
- Define several functions ("new_file()", "open_file()", "save_file()", "cut_text()", "copy_text()", "paste_text()", and "about()") that will be executed when submenu items are clicked. You can replace print statements with actual functionality.
- Create the main window using tk.Tk() and set its title.
- Create a menu bar using Menu(root) and set it as the main menu using root.config(menu=menu_bar).
- Create three menus: 'File', 'Edit', and 'Help', using Menu(menu_bar, tearoff=0). The tearoff option specifies whether the menu can be torn off as a separate window (0 means no).
- Add submenu items to each menu using menu.add_command(label="Item Label", command=callback_function). Label specifies the item's label, and command specifies the action to take when the item is clicked.
- Use menu_bar.add_cascade(label="Menu Name", menu=menu) to add each menu to the menu bar.
- The main event loop, root.mainloop(), starts the GUI application.
Sample Output:
New File Cut Text About this application![]()
Flowchart:
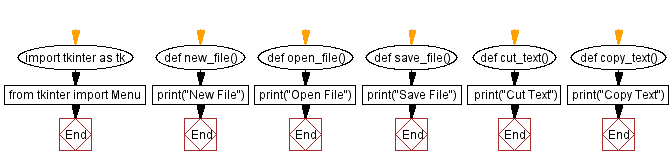
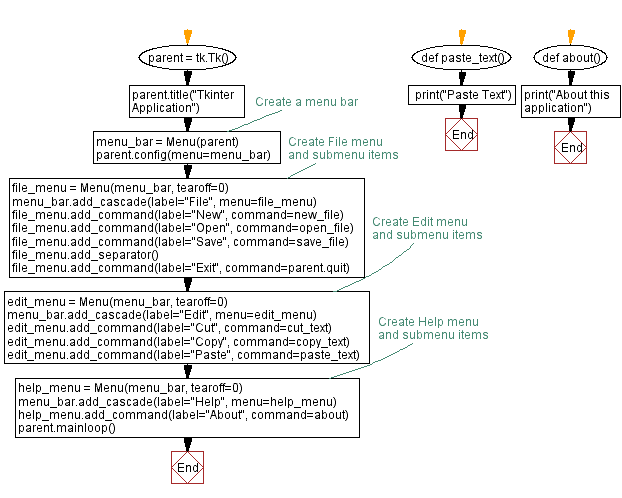
Python Code Editor:
Previous: Building a Hierarchical list widget.
Next: Font size control with Tkinter scale widget.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/tkinter/python-tkinter-widgets-exercise-15.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics