Python GUI Program: Font size control with Tkinter scale widget
Write a Python GUI program to create a Scale widget that controls the font size of a label using tkinter module.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import ttk
def update_font_size(value):
try:
new_font_size = int(float(value))
label.config(font=("Roboto", new_font_size))
except ValueError:
pass # Ignore non-integer values
parent = tk.Tk()
parent.title("Font Size Control")
# Create a Scale widget for font size control
font_size_scale = ttk.Scale(parent, from_=10, to=36, orient="horizontal", length=200, command=update_font_size)
font_size_scale.pack(pady=10)
# Create a label with initial font size
initial_font_size = 16
label = ttk.Label(parent, text="Font Size", font=("Roboto", initial_font_size))
label.pack(pady=10)
parent.mainloop()
Explanation:
In the exercise above -
- First we create a Tkinter main window using tk.Tk() and set its title.
- Next we create a ttk.Scale widget named 'font_size_scale' that allows users to select font sizes from 10 to 36.
- Create a label widget named label with an initial font size of 16 and display the text "Font Size."
- The "update_font_size()" function updates the font size of the label based on the value of the Scale widget. It converts the value to an integer and sets the font size accordingly.
- A Scale widget and a Label widget are packed into the main window.
- The main event loop, root.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
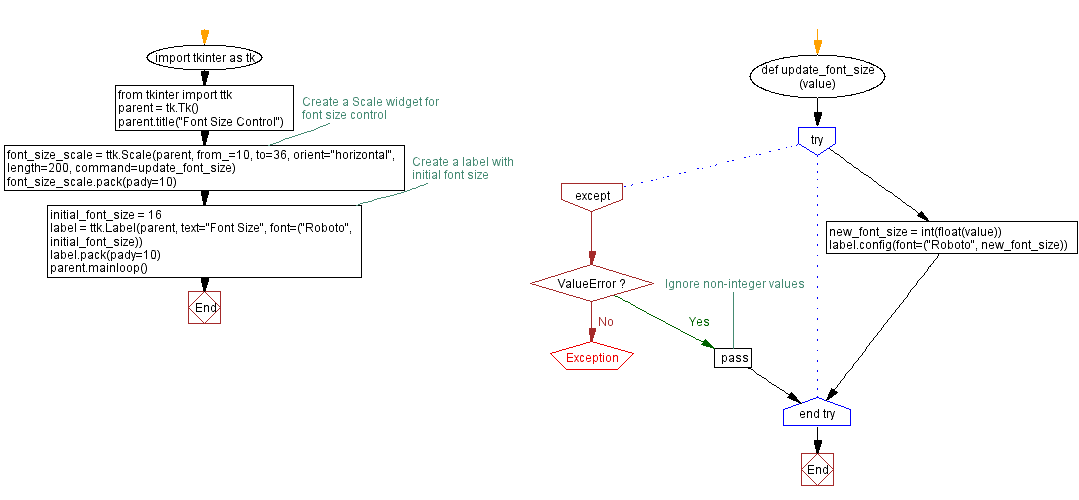
Python Code Editor:
Previous: Creating a menu bar with Tkinter.
Next: Selecting dates made easy.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics