Python Tkinter date Entry widget: Selecting dates made easy
Python tkinter widgets: Exercise-17 with Solution
Write a Python GUI program to create a Date Entry widget for selecting a date using tkinter module.
Sample Solution:
Python Code:
import tkinter as tk
from tkcalendar import DateEntry
def get_selected_date():
selected_date = cal.get_date()
selected_date_label.config(text=f"Selected Date: {selected_date}")
parent = tk.Tk()
parent.title("Date Entry Example")
# Create a Date Entry widget
cal = DateEntry(parent, width=12, background="darkblue", foreground="white", borderwidth=2)
cal.pack(padx=10, pady=10)
# Create a button to get the selected date
get_date_button = tk.Button(parent, text="Get Selected Date", command=get_selected_date)
get_date_button.pack(pady=10)
# Create a label to display the selected date
selected_date_label = tk.Label(parent, text="", font=("Helvetica", 12))
selected_date_label.pack()
parent.mainloop()
Explanation:
In the exercise above -
- Import tkinter as tk and DateEntry from tkcalendar.
- Define a function "get_selected_date()" that retrieves the selected date from the DateEntry widget and updates a label to display the selected date.
- Create the main window using tk.Tk() and set its title.
- Create a DateEntry widget named cal, configure its appearance, and pack it into the main window.
- Create a button labeled "Get Selected Date" and associate it with the get_selected_date function to retrieve and display the selected date.
- Create a label named 'selected_date_label' that will display the selected date.
- The main event loop, parent.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
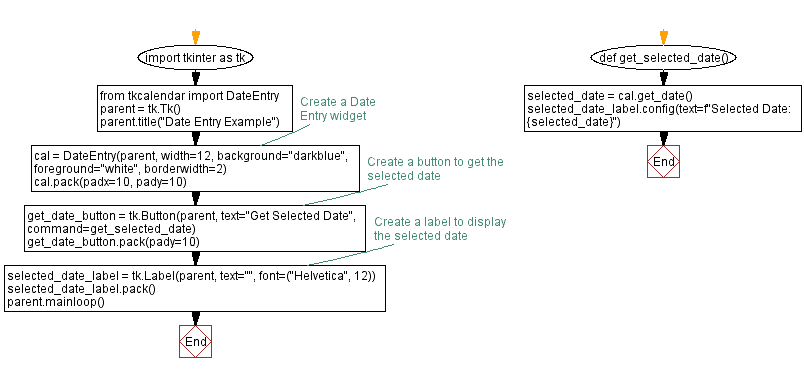
Python Code Editor:
Previous: Font size control with Tkinter scale widget.
Next: Sorting and columns made easy.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/tkinter/python-tkinter-widgets-exercise-17.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics