Python Tkinter progress bar: Dynamic percentage updates
Write a Python GUI program to create a Progress bar widget that updates based on a given percentage using tkinter module.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import ttk
def update_progress():
new_value = progress_var.get()
new_value += 10
if new_value > 100:
new_value = 0
progress_var.set(new_value)
progress_bar["value"] = new_value
parent.after(1000, update_progress) # Update every 1000 milliseconds (1 second)
parent = tk.Tk()
parent.title("Progress Bar Example")
# Create a Progress Bar widget
progress_var = tk.DoubleVar()
progress_bar = ttk.Progressbar(parent, variable=progress_var, maximum=100)
progress_bar.pack(padx=20, pady=20, fill="x")
# Create a button to update the progress bar
update_button = tk.Button(parent, text="Update Progress", command=update_progress)
update_button.pack()
parent.after(1000, update_progress) # Start updating the progress bar
parent.mainloop()
Explanation:
In the exercise above -
- Import 'tkinter' as 'tk' and 'ttk' for creating GUI components.
- Define a function "update_progress()" that updates the progress bar's value. It increments the progress by 10 units and resets it to 0 when it reaches 100. The "after()" method schedules the next update after 1000 milliseconds (1 second).
- Create the main window using tk.Tk() and set its title.
- Create a DoubleVar named 'progress_var' to hold the progress bar's value.
- Create a Progress Bar widget named 'progress_bar' and associate it with progress_var. The maximum parameter sets the progress bar maximum value to 100.
- Create a button named 'update_button' that, when clicked, calls the 'update_progress' function to update the progress bar.
- We use the "after()" method to update the progress bar every 1000 milliseconds (1 second).
- The main event loop, parent.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
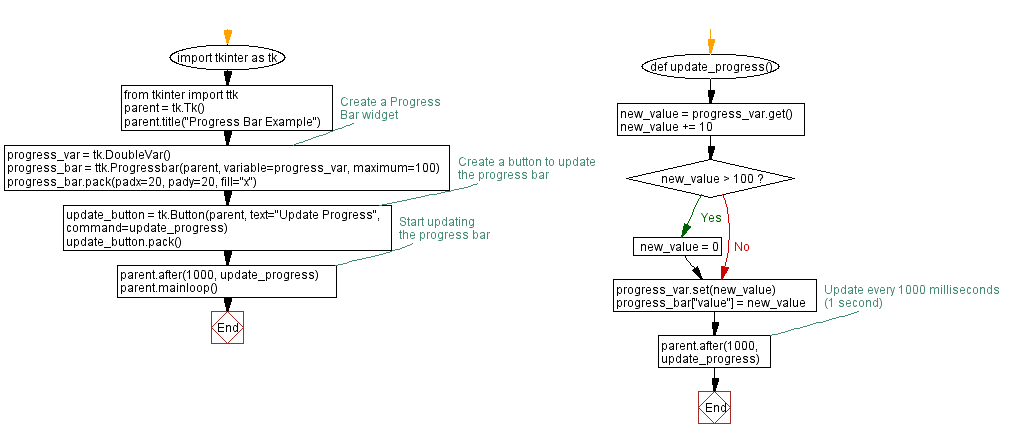
Python Code Editor:
Previous: Sorting and columns made easy.
Next: Custom range and step size made easy.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics