Python tkinter widgets Exercise: Add a canvas in your application using tkinter module
Write a Python GUI program to add a canvas in your application using tkinter module.
Sample Solution:
Python Code:
import tkinter as tk
parent = tk.Tk()
canvas_width = 100
canvas_height = 80
w = tk.Canvas(parent,
width=canvas_width,
height=canvas_height)
w.pack()
y = int(canvas_height / 2)
w.create_line(0, y, canvas_width, y, fill="#476042")
parent.mainloop()
Explanation:
In the exercise above -
- import tkinter as tk - Import the required libraries.
- parent = tk.Tk() - Create the main Tkinter window.
- canvas_width = 100 canvas_height = 80 - Define canvas dimensions.
- w = tk.Canvas(parent, width=canvas_width, height=canvas_height) - Create a Canvas widget within the main window.
- y = int(canvas_height / 2) - Calculate the y-coordinate for the horizontal line.
- w.create_line(0, y, canvas_width, y, fill="#476042") - Create a horizontal line on the canvas.
- parent.mainloop() - Start the Tkinter main loop.
Sample Output:
Flowchart:
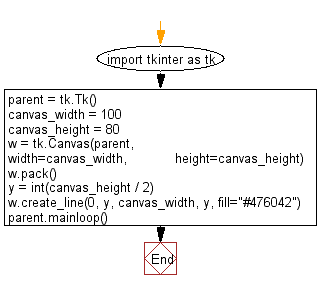
Go to:
Previous: Write a Python GUI program to add a button in your application using tkinter module.
Next: Write a Python GUI program to create two buttons exit and hello using tkinter module.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.