Python Tkinter Colorchooser: Effortlessly choose and display colors
Write a Python GUI program to create a Colorchooser widget to select a color using tkinter module.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import colorchooser
def choose_color():
color = colorchooser.askcolor(title="Select a Color")
if color[1]:
selected_color_label.config(text=f"Selected Color: {color[1]}")
color_frame.config(bg=color[1])
parent = tk.Tk()
parent.title("Colorchooser Example")
# Create a button to open the color chooser dialog
choose_color_button = tk.Button(parent, text="Choose a Color", command=choose_color)
choose_color_button.pack(padx=20, pady=20)
# Create a label to display the selected color
selected_color_label = tk.Label(parent, text="Selected Color:", font=("Helvetica", 10))
selected_color_label.pack()
# Create a frame to display the selected color
color_frame = tk.Frame(parent, width=100, height=50)
color_frame.pack(padx=20, pady=20)
parent.mainloop()
Explanation:
In the exercise above -
- Import 'tkinter' as 'tk' and 'colorchooser' for creating GUI components and accessing the color chooser dialog.
- Define a function "choose_color()" that opens the color chooser dialog using colorchooser.askcolor(). As soon as a color is selected and the dialog is closed, it returns a tuple containing the color's RGB format and its name. We update the label and frame with the selected color.
- Create the main window using tk.Tk() and set its title.
- The 'choose_color_button' button will call the "choose_color()" function when clicked.
- Create a label named 'selected_color_label' to display the selected color.
- Create a frame named 'color_frame' to display the selected color as a background color.
- The main event loop, root.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
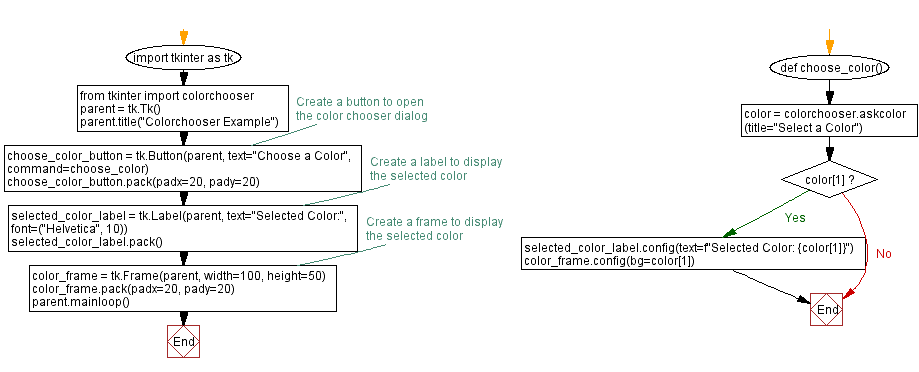
Go to:
Previous: Multi-line text with word wrapping made easy.
Next: Python Tkinter dialogs and File handling Exercises Home
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.