Python tkinter widgets Exercise: Create three single line text-box to accept a value from the user using tkinter module
Write a Python GUI program to create three single line text-box to accept a value from the user using tkinter module.
Sample Solution:
Python Code:
import tkinter as tk
parent = tk.Tk()
parent.geometry("400x250")
name = tk.Label(parent, text = "Name").place(x = 30, y = 50)
email = tk.Label(parent, text = "User ID").place(x = 30, y = 90)
password = tk.Label(parent, text = "Password").place(x = 30, y = 130)
sbmitbtn = tk.Button(parent, text = "Submit", activebackground = "green", activeforeground = "blue").place(x = 120, y = 170)
entry1 = tk.Entry(parent).place(x = 85, y = 50)
entry2 = tk.Entry(parent).place(x = 85, y = 90)
entry3 = tk.Entry(parent).place(x = 90, y = 130)
parent.mainloop()
Explanation:
In the exercise above -
- import tkinter as tk - Import the Tkinter library (tkinter).
- parent = tk.Tk() - Create the main Tkinter window (parent)
- parent.geometry("400x250") - Set its initial size to 400x250 pixels.
- Create Label widgets (name, email, password) to display text ("Name," "User ID," "Password") at specific positions within the window.
- sbmitbtn = tk.Button(parent, text = "Submit", activebackground = "green", activeforeground = "blue").place(x = 120, y = 170) - Create a Submit Button (sbmitbtn) with custom active background and foreground colors. Use the place() method to specify the exact x and y coordinates for placing each widget.
- Create Entry widgets (entry1, entry2, entry3) for user input.
- parent.mainloop() - Start the Tkinter main loop to run the GUI application.
Sample Output:
Flowchart:
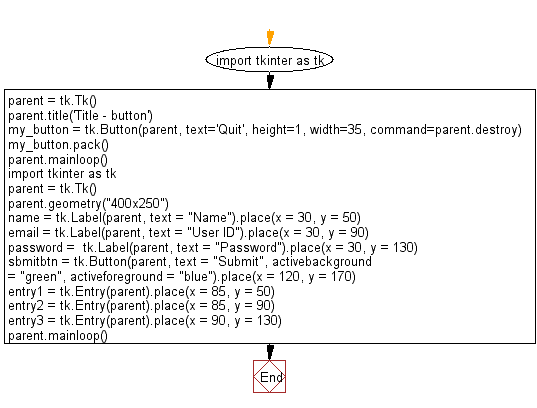
Go to:
Previous: Write a Python GUI program to create a Text widget using tkinter module.Insert a string at the beginning then insert a string into the current text. Delete the first and last character of the text.
Next: Write a Python GUI program to create three radio buttons widgets using tkinter module.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.