Python Exercise: Unzip a list of tuples into individual lists
17. Unzip a List of Tuples into Individual Lists
Write a Python program to unzip a list of tuples into individual lists.
Sample Solution:
Python Code:
# Create a list of tuples, where each tuple contains two elements.
l = [(1, 2), (3, 4), (8, 9)]
# Use the 'zip' function with the '*' operator to unpack and zip the tuples.
# This creates new tuples where the first elements from the original tuples are combined into one tuple,
# and the second elements from the original tuples are combined into another tuple.
result = list(zip(*l))
# Print the result, which is a list of two tuples formed by zipping the original tuples.
print(result)
Sample Output:
[(1, 3, 8), (2, 4, 9)]
Flowchart:
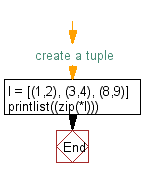
For more Practice: Solve these Related Problems:
- Write a Python program to unzip a list of tuples into separate lists using the zip() function with unpacking.
- Write a Python program to implement a function that takes a list of tuples and returns individual lists for each tuple element.
- Write a Python program to use list comprehension with zip(*) to extract columns from a list of tuples.
- Write a Python program to demonstrate unzipping by converting a list of tuples into a tuple of lists.
Go to:
Previous: Write a Python program to convert a tuple to a dictionary.
Next: Write a Python program to reverse a tuple.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.