Python Exercise: Sort a tuple by its float element
23. Sort a Tuple by Its Float Element
Write a Python program to sort a tuple by its float element.
Sample Solution:
Python Code:
# Create a list of tuples 'price', where each tuple represents an item and its price as a string.
price = [('item1', '12.20'), ('item2', '15.10'), ('item3', '24.5')]
# Sort the 'price' list based on the price values (the second element in each tuple).
# The 'key' argument specifies a lambda function to convert the price strings to float values.
# Sorting is done in reverse (descending) order using the 'reverse' argument.
sorted_price = sorted(price, key=lambda x: float(x[1]), reverse=True)
# Print the 'sorted_price' list, which contains the items sorted by price in descending order.
print(sorted_price)
Sample Output:
[('item3', '24.5'), ('item2', '15.10'), ('item1', '12.20')]
Flowchart:
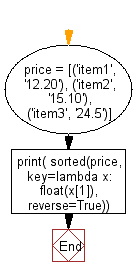
For more Practice: Solve these Related Problems:
- Write a Python program to sort a tuple of tuples by the float conversion of their second element.
- Write a Python program to use sorted() with a lambda function to order tuples based on a float value in one of the positions.
- Write a Python program to implement a function that returns a sorted tuple based on the numerical value of one element.
- Write a Python program to compare two tuples based on the float conversion of a specific element and sort them accordingly.
Go to:
Previous: Write a Python program to remove an empty tuple(s) from a list of tuples.
Next: Write a Python program to count the elements in a list until an element is a tuple.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.