Python Exercise: Convert a tuple of string values to a tuple of integer values
28. Convert a Tuple of String Values to a Tuple of Integer Values
Write a Python program to convert a tuple of string values to a tuple of integer values.
Sample Solution:
Python Code:
# Define a function named 'tuple_int_str' that takes a tuple of tuples 'tuple_str' as input.
def tuple_int_str(tuple_str):
# Create a new tuple 'result' by converting the string elements in each inner tuple to integers.
result = tuple((int(x[0]), int(x[1])) for x in tuple_str)
# Return the resulting tuple.
return result
# Create a tuple of tuples 'tuple_str' containing pairs of strings.
tuple_str = (('333', '33'), ('1416', '55'))
# Print a message indicating the original tuple values.
print("Original tuple values:")
# Print the 'tuple_str' tuple.
print(tuple_str)
# Print a message indicating the new tuple values, which are obtained by converting the strings to integers using the 'tuple_int_str' function.
print("\nNew tuple values:")
# Call the 'tuple_int_str' function to convert the strings to integers and print the result.
print(tuple_int_str(tuple_str))
Sample Output:
Original tuple values: (('333', '33'), ('1416', '55')) New tuple values: ((333, 33), (1416, 55))
Flowchart:
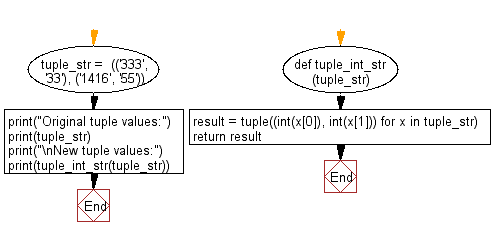
For more Practice: Solve these Related Problems:
- Write a Python program to convert a tuple of numeric strings into a tuple of integers using tuple comprehension.
- Write a Python program to iterate over a tuple and cast each string element to an integer, then output the new tuple.
- Write a Python program to use map() to convert a tuple of strings to integers and then convert the result back to a tuple.
- Write a Python program to implement a function that takes a tuple of strings and returns a tuple of numbers.
Go to:
Previous: Write a Python program to calculate the average value of the numbers in a given tuple of tuples.
Next: Write a Python program to convert a given tuple of positive integers into an integer.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.