Python Exercise: Compute element-wise sum of given tuples
Write a Python program to compute the element-wise sum of given tuples.
Sample Solution:
Python Code:
# Define three tuples 'x', 'y', and 'z' with integer elements.
x = (1, 2, 3, 4)
y = (3, 5, 2, 1)
z = (2, 2, 3, 1)
# Print a message indicating the original lists of tuples.
print("Original lists:")
print(x)
print(y)
print(z)
# Print a message indicating the element-wise sum of the said tuples.
# Use the 'zip' function to pair corresponding elements from 'x', 'y', and 'z' tuples, and then use 'map' and 'sum' to calculate the element-wise sum.
result = tuple(map(sum, zip(x, y, z)))
# Print the 'result' tuple containing the element-wise sum.
print("\nElement-wise sum of the said tuples:")
print(result)
Sample Output:
Original lists: (1, 2, 3, 4) (3, 5, 2, 1) (2, 2, 3, 1) Element-wise sum of the said tuples: (6, 9, 8, 6)
Flowchart:
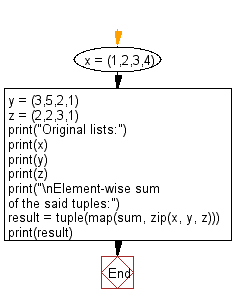
Python Code Editor:
Previous: Write a Python program to check if a specified element presents in a tuple of tuples.
Next: Write a Python program to compute the sum of all the elements of each tuple stored inside a list of tuples.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics