Python unit test: Check equality of two lists
3. Unit Test for Equality of Two Lists
Write a Python unit test program that checks if two lists are equal.
Sample Solution:
Python Code:
# Import the 'unittest' module for writing unit tests.
import unittest
# Define a function 'lists_are_equal' to check if two lists are equal.
def lists_are_equal(nums1, nums2):
return nums1 == nums2
# Define a test case class 'TestListsEquality' that inherits from 'unittest.TestCase'.
class TestListsEquality(unittest.TestCase):
# Define a test method 'test_equal_lists' to test equal lists.
def test_equal_lists(self):
# Define two lists 'nums1' and 'nums2' for testing equal or unequal lists.
nums1 = [10, 20, 30, 40]
nums2 = [10, 20, 30, 40]
# Uncomment one pair of 'nums1' and 'nums2' for testing equal or unequal lists.
#nums1 = [10, 20, 30, 40]
#nums2 = [10, 20, 30, 50]
print("\nEqual list test:\n", nums1, "\n", nums2)
# Assert that the lists are equal.
self.assertTrue(lists_are_equal(nums1, nums2), "The lists are not equal")
# Define a test method 'test_unequal_lists' to test unequal lists.
def test_unequal_lists(self):
# Define two lists 'nums1' and 'nums2' for testing equal or unequal lists.
nums1 = [10, 20, 30, 40]
nums2 = [30, 20, 10, 40]
# Uncomment one pair of 'nums1' and 'nums2' for testing equal or unequal lists.
#nums1 = [10, 20, 30, 40]
#nums2 = [10, 20, 30, 40]
print("\nUnequal list test:\n", nums1, "\n", nums2)
# Assert that the lists are not equal.
self.assertFalse(lists_are_equal(nums1, nums2), "The lists are equal")
# Check if the script is run as the main program.
if __name__ == '__main__':
# Run the test cases using 'unittest.main()'.
unittest.main()
Sample Output:
Ran 2 tests in 0.001s OK Equal list test: [10, 20, 30, 40] [10, 20, 30, 40] Unequal list test: [10, 20, 30, 40] [30, 20, 10, 40]
Ran 2 tests in 0.001s FAILED (failures=2) Equal list test: [10, 20, 30, 40] [10, 20, 30, 50] Unequal list test: [10, 20, 30, 40] [10, 20, 30, 40]
Explanation:
In the above exercise, we have a function lists_are_equal(nums1, nums2) that takes two lists as input and returns True if the lists are equal, and False otherwise. The TestListsEquality class inherits from unittest.TestCase, and we define two test methods: test_equal_lists and test_unequal_lists.
Flowchart:
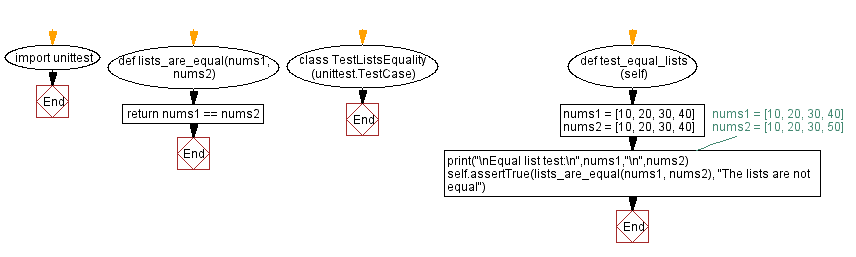
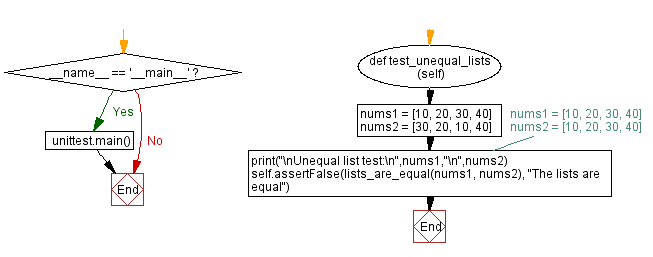
For more Practice: Solve these Related Problems:
- Write a Python unit test program using unittest’s assertListEqual to check if two lists with identical elements (and order) are equal.
- Write a Python unit test program to compare two lists with nested lists for equality.
- Write a Python unit test program that verifies that two lists are not equal if they differ in length or order.
- Write a Python unit test program to test a custom function that compares lists element by element and returns a boolean for equality.
Go to:
Previous: Check list sorting.
Next: Check palindrome string.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.