Implementing Retry Mechanism in Python urllib3 for Resilient HTTP Requests
Python urllib3 : Exercise-11 with Solution
Write a Python program that constructs a URL with query parameters and ensures that the URL is encoded correctly.
Sample Solution:
Python Code :
# Import the urllib3 library
import urllib3
# Define the base URL without query parameters
base_url = 'https://example.com/api'
# Define the query parameters as a dictionary
query_params = {
'param1': 'value1',
'param2': 'value2',
'param3': 'special character: $',
}
# Use the urllib3 urlencode function to encode the query parameters
encoded_params = urllib3.request.urlencode(query_params)
# Combine the base URL and encoded query parameters to form the complete URL
complete_url = f"{base_url}?{encoded_params}"
# Print the complete URL
print(f"Complete URL: {complete_url}")
Sample Output:
Complete URL: https://example.com/api?param1=value1¶m2=value2¶m3=special+character%3A+%24
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- Import the urllib3 library: Imports the "urllib3" library, which is a powerful tool for handling HTTP requests in Python.
- Define the base URL without query parameters: Sets a base URL (e.g., 'https://example.com/api') without any query parameters. This is the starting point for constructing the complete URL.
- Define the query parameters as a dictionary: Create a dictionary (query_params) with key-value pairs representing the query parameters that will be appended to the base URL.
- Use the urllib3 urlencode function to encode the query parameters: Utilize the urllib3.request.urlencode function to encode the dictionary of query parameters into a URL-encoded string. This step ensures that special characters are properly handled.
- Combine the base URL and encoded query parameters to form the complete URL: Concatenates the base URL and the encoded query parameters using the f"{base_url}?{encoded_params}" syntax. This creates a complete URL with the specified query parameters.
- Print the complete URL: Outputs the generated complete URL to the console for verification purposes.
Flowchart:
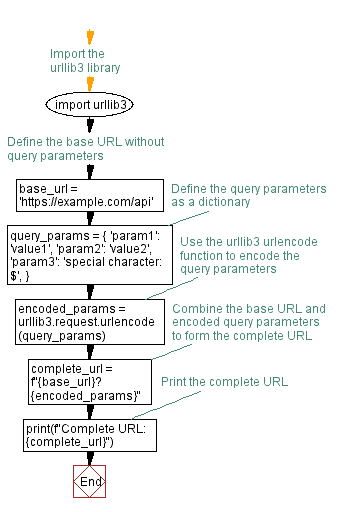
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python Large File Download with Stream Parameter example.
Next: Logging HTTP Requests in Python urllib3: Understanding Request Hooks.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/urllib3/python-urllib3-exercise-11.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics