Making API Requests with digest authentication in Python urllib3
Python urllib3 : Exercise-13 with Solution
Write a Python program that makes a request to an API that requires Digest Authentication.
Sample Solution:
Python Code :
# Import necessary libraries
import requests
from requests.auth import HTTPDigestAuth
import urllib3
# Create a custom urllib3 PoolManager
http = urllib3.PoolManager()
# API endpoint that requires Digest Authentication
api_url = 'https://example.com/api/endpoint' # Replace with the actual API endpoint
# Username and password for Digest Authentication
username = 'your_username'
password = 'your_password'
# Make a request with Digest Authentication using requests library
response = requests.get(api_url, auth=HTTPDigestAuth(username, password))
# Check the response
if response.status_code == 200:
print("Request successful:")
print(response.text)
else:
print(f"Error: Unable to fetch data. Status Code: {response.status_code}")
# Close the urllib3 connection pool
http.clear()
Sample Output:
Error: Unable to fetch data. Status Code: 404
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- urllib3.PoolManager() is used to create a custom connection pool manager.
- api_url should be replaced with the actual API endpoint that requires Digest Authentication.
- 'username' and 'password' are the credentials for Digest Authentication.
- The "requests.get" method is used to make a GET request with Digest Authentication.
- If the response status code is 200, the response content is printed. Otherwise, an error message is printed.
Flowchart:
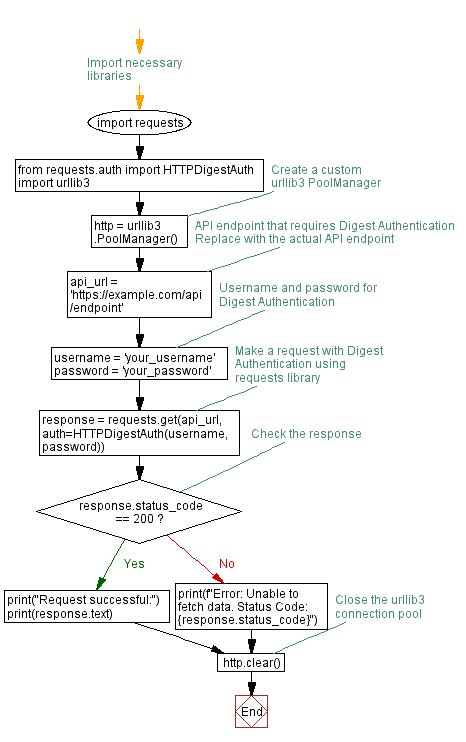
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Logging HTTP Requests in Python urllib3: Understanding Request Hooks.
Next: Creating a session for multiple Requests with Python urllib3 PoolManager.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/urllib3/python-urllib3-exercise-13.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics