Implementing a Custom SSL Context for Secure HTTPS requests in Python urllib3
Write a Python program that implements a custom SSL context for handling specific SSL configurations.
Sample Solution:
Python Code :
# Import necessary libraries
import urllib3
import ssl
# Create a custom SSL context with specific configurations
custom_ssl_context = ssl.create_default_context()
# Set custom SSL options if needed (e.g., disable SSL verification)
# custom_ssl_context.options &= ~ssl.OP_NO_SSLv3
# Create a urllib3 PoolManager with the custom SSL context
http = urllib3.PoolManager(cert_reqs='CERT_REQUIRED', ssl_context=custom_ssl_context)
# Define the URL for the HTTPS request
url = 'https://www.example.com' # Replace with the actual URL
# Make an HTTPS GET request using the custom SSL context
try:
response = http.request('GET', url)
# Check if the request was successful (status code 200)
if response.status == 200:
print("Request Successful:")
print(response.data.decode('utf-8'))
else:
print(f"Error: Unable to fetch data. Status Code: {response.status}")
except urllib3.exceptions.RequestError as e:
print(f"Error: {e}")
# Clear the urllib3 connection pool
http.clear()
Sample Output:
Request Successful: <!doctype html> <html> <head> <title>Example Domain</title> <meta charset="utf-8" /> <meta http-equiv="Content-type" content="text/html; charset=utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <style type="text/css"> body { background-color: #f0f0f2; margin: 0; padding: 0; font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif; } div { width: 600px; margin: 5em auto; padding: 2em; background-color: #fdfdff; border-radius: 0.5em; box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02); } a:link, a:visited { color: #38488f; text-decoration: none; } @media (max-width: 700px) { div { margin: 0 auto; width: auto; } } </style> </head> <body> <div> <h1>Example Domain</h1> <p>This domain is for use in illustrative examples in documents. You may use this domain in literature without prior coordination or asking for permission.</p> <p><a href="https://www.iana.org/domains/example">More information...</a></p> </div> </body> </html>
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- Import Libraries: Import the necessary libraries, including "urllib3" for making HTTP requests and "ssl" for creating a custom SSL context.
- Create Custom SSL Context: Use ssl.create_default_context() to create a custom SSL context. You can set additional SSL options within the 'custom_ssl_context' variable, based on your specific requirements.
- Create urllib3 PoolManager: Create an instance of "urllib3.PoolManager" with the custom SSL context. The cert_reqs='CERT_REQUIRED' parameter enforces certificate verification.
- Define Request URL: Specify the URL for the HTTPS request. Replace 'https://www.example.com' with your actual URL.
- Make HTTPS Request: Use the "http.request" method to make an HTTPS GET request to the specified URL. Handle the response accordingly, checking for a successful status code (200).
- Exception Handling: Catch and handle "urllib3.exceptions.RequestError" exceptions that might occur during the request.
- Clear Connection Pool: After making the request, clear the urllib3 connection pool using "http.clear().
Flowchart:
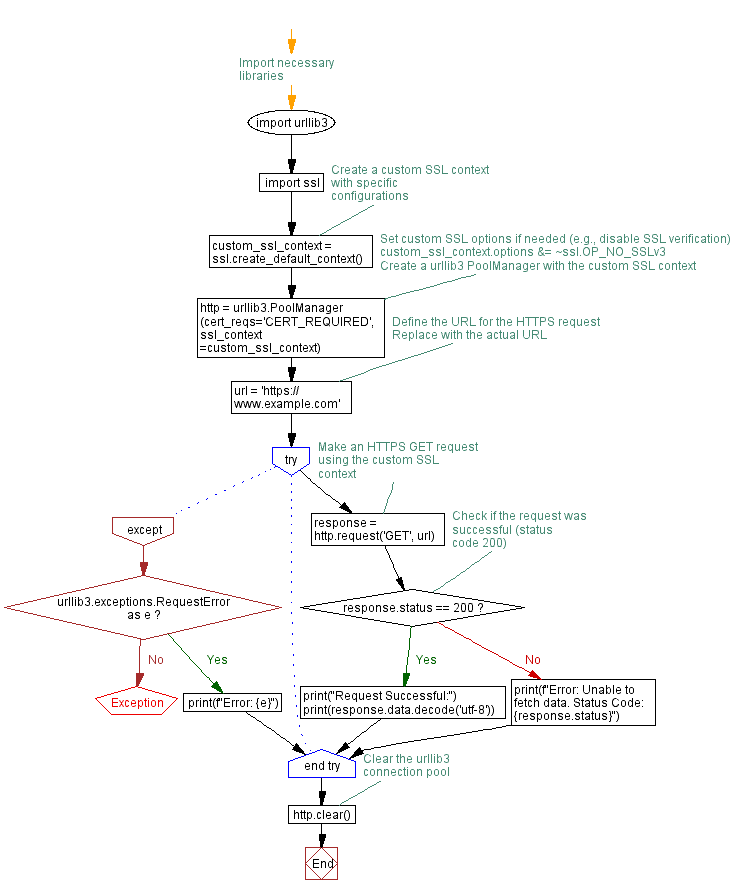
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Making API Requests with digest authentication in Python urllib3.
Next: Implementing Retry Mechanism in Python urllib3 for Resilient HTTP Requests.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics