Python urllib3: Demonstrate Cookie persistence for seamless Web requests
Write a Python program that sends and receives cookies with urllib3, demonstrating cookie persistence.
Sample Solution:
Python Code :
import urllib3
# Create a PoolManager
http = urllib3.PoolManager()
# Step 1: Initial Request
initial_url = 'https://example.com/initial'
initial_response = http.request('GET', initial_url)
# Step 2: Extract Cookies from Initial Response
initial_cookies = initial_response.headers.get('Set-Cookie')
# Step 3: Persist Cookies (store in a dictionary, for example)
persisted_cookies = {'cookie_name': 'cookie_value'}
# Step 4: Subsequent Request with Persisted Cookies
subsequent_url = 'https://example.com/subsequent'
subsequent_headers = {'Cookie': '; '.join([f'{name}={value}' for name, value in persisted_cookies.items()])}
subsequent_response = http.request('GET', subsequent_url, headers=subsequent_headers)
# Step 5: Receive and Print Response
print(subsequent_response.data.decode('utf-8'))
Sample Output:
<!doctype html> <html> <head> <title>Example Domain</title> <meta charset="utf-8" /> <meta http-equiv="Content-type" content="text/html; charset=utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <style type="text/css"> body { background-color: #f0f0f2; margin: 0; padding: 0; font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif; } div { width: 600px; margin: 5em auto; padding: 2em; background-color: #fdfdff; border-radius: 0.5em; box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02); } a:link, a:visited { color: #38488f; text-decoration: none; } @media (max-width: 700px) { div { margin: 0 auto; width: auto; } } </style> </head> <body> <div> <h1>Example Domain</h1> <p>This domain is for use in illustrative examples in documents. You may use this domain in literature without prior coordination or asking for permission.</p> <p><a href="https://www.iana.org/domains/example">More information...</a></p> </div> </body> </html>
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- Import the Necessary Modules:
- Import the "urllib3" module.
- Create a PoolManager Object:
- Create a "PoolManager" object to manage the connection pool for making HTTP requests.
- Send a Request with Cookies:
- Make an initial GET request to a URL and receive a response.
- Extract cookies from response headers.
- Persist Cookies:
- Store the received cookies in a data structure (e.g., a dictionary) for persistence.
- Send a Subsequent Request with Persisted Cookies:
- Make another request to the same or another URL, including the persisted cookies in the request headers.
- Receive and Print the Response:
- Receive the response and print the content to verify successful communication with persisted cookies.
Flowchart:
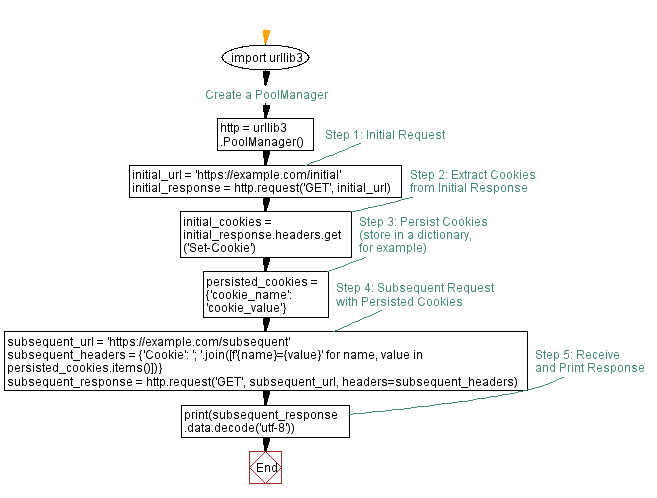
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python file upload with urllib3: Making POST Requests simplified.
Next: Python File Upload: Simulate POST request with multipart/Form-Data.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics