Python Program: Observing urllib3 Response compression handling
Write a Python program that requests a server that supports response compression (gzip or deflate), and observe how urllib3 handles it.
Sample Solution:
Python Code :
import urllib3
# Create a PoolManager
http = urllib3.PoolManager()
# Define the URL of the server that supports response compression
url = 'https://httpbin.org/gzip'
try:
# Make a GET request
response = http.request('GET', url)
# Check if the request was successful (status code 200)
if response.status == 200:
# Print the content of the response
print("Response Content:")
print(response.data.decode('utf-8'))
else:
print(f"Error: Unable to fetch data. Status Code: {response.status}")
except Exception as e:
print(f"Error: {e}")
Sample Output:
Response Content: { "gzipped": true, "headers": { "Accept-Encoding": "identity", "Host": "httpbin.org", "User-Agent": "python-urllib3/1.26.16", "X-Amzn-Trace-Id": "Root=1-65c39992-23574c3474d0f427038091a2" }, "method": "GET", "origin": "103.51.149.158" }
Explanation:
In the above exercise,
- We import the urllib3 module.
- We create a PoolManager object to manage the connection pool for making HTTP requests.
- We define the URL of the server that supports response compression. Here, we use "https://httpbin.org/gzip", which is a server that responds with gzipped content.
- We make a GET request to the server using http.request('GET', url).
- We decode and print the content of the response if the request was successful.
Flowchart:
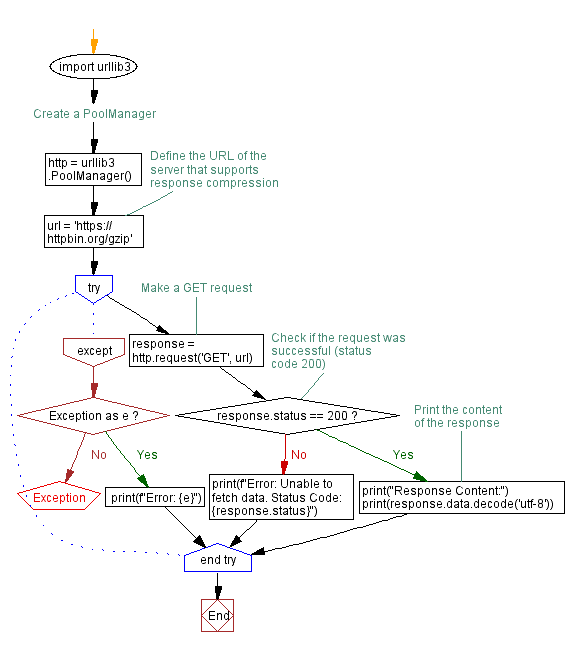
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python Program: Maximum connection Pool size analysis.
Next: Python Program: Custom Redirect Handling with urllib3.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.