Python Web Scraping: Get the number of following on a Twitter account
Write a Python program to get the number of "following" on a Twitter account.
Sample Solution:
Python Code:
from bs4 import BeautifulSoup
import requests
handle = input('Input your account name on Twitter: ')
temp = requests.get('https://twitter.com/'+handle)
bs = BeautifulSoup(temp.text,'lxml')
try:
following_box = bs.find('li',{'class':'ProfileNav-item ProfileNav-item--following'})
following = following_box.find('a').find('span',{'class':'ProfileNav-value'})
print("{} is following {} people.".format(handle,following.get('data-count')))
except:
print('Account name not found...')
Sample Output:
Input your account name on Twitter: @prasanta @prasanta is following 110 people.
Flowchart:
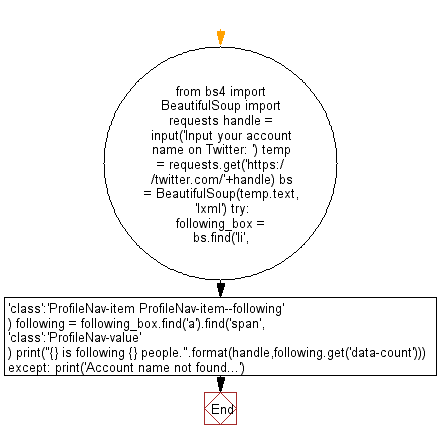
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to get the number of followers of a given twitter account.
Next: Write a Python program to get the number of post on Twitter liked by a given account.
What is the difficulty level of this exercise?