Python Web Scraping: Display the date, days, title, city, country of next 25 Hackevents
Write a Python program to display the date, days, title, city, country of next 25 Hackevents.
Sample Solution:
Python Code:
import requests
from bs4 import BeautifulSoup
res = requests.get('https://hackevents.co/hackathons')
bs = BeautifulSoup(res.text, 'lxml')
hacks_data = bs.find_all('div',{'class':'hackathon '})
for i,f in enumerate(hacks_data,1):
hacks_month = f.find('div',{'class':'date'}).find('div',{'class':'date-month'}).text.strip()
hacks_date = f.find('div',{'class':'date'}).find('div',{'class':'date-day-number'}).text.strip()
hacks_days = f.find('div',{'class':'date'}).find('div',{'class':'date-week-days'}).text.strip()
hacks_final_date = "{} {}, {} ".format(hacks_date, hacks_month, hacks_days )
hacks_name = f.find('div',{'class':'info'}).find('h2').text.strip()
hacks_city = f.find('div',{'class':'info'}).find('p').find('span',{'class':'city'}).text.strip()
hacks_country = f.find('div',{'class':'info'}).find('p').find('span',{'class':'country'}).text.strip()
print("{:<5}{:<15}: {:<90}: {}, {}\n ".format(str(i)+')',hacks_final_date, hacks_name.title(), hacks_city, hacks_country))
Sample Output:
1) 7 Jul, Sat-Sun : Angelhack Cyprus Hackathon 2018 : Limassol, Cyprus 2) 10 Jul, Tue : Network Resilience Hackathon : Leeds, United Kingdom 3) 10 Jul, Tue : Découvrir Le Hacking Silver Camp : Strasbourg, France 4) 10 Jul, Tue-Wed : Hack4Tk Thyssenkrupp Hackathon 2018 : Essen, Germany 5) 10 Jul, Tue : Hackathon For Supply Chain Transparency With Zalando Se And Volkswagen Group : Berlin, Germany 6) 11 Jul, Wed-Fri : Clean Water Hackathon : Kensington, Australia 7) 11 Jul, Wed : Hack|Bay 2018 Evening Gala : Nürnberg, Germany 8) 11 Jul, Wed : Society Of Medical Innovation: Health Hackathon : Kensington, Australia 9) 12 Jul, Thu-Thu : Atlantic Lottery Hackathon 007: Space Lottery : Halifax, Canada 10) 12 Jul, Thu : Vodw Brussels - Digital Design Hackaton : Brussel, Belgium 11) 12 Jul, Thu : Düsseldorf: Dci Hackathon : Düsseldorf, Germany 12) 12 Jul, Thu-Fri : Aasqa Software Testing Hackathon : Bentley, Australia 13) 12 Jul, Thu-Fri : Voice Assistants For All Hackathon : Los Angeles, USA 14) 12 Jul, Thu : Crowdfunding Hackathon Barcelona : Barcelona, Spain 15) 12 Jul, Thu-Sat : Cross-Industry Ai Hack Powered By Bmw & Siemens : Munich, Germany 16) 13 Jul, Fri : Lead4Hope - Hackney For Social Change : Shoreditch, United Kingdom 17) 13 Jul, Fri-Sun : Code For Cognitive Aid (Hackathon/Makeathon/Stem) : Portola Valley, USA 18) 13 Jul, Fri : Lead4Hope - Hackney For Social Change : London, United Kingdom 19) 13 Jul, Fri-Sun : Hack For Venezuela Hackathon : New York, USA 20) 13 Jul, Fri-Sun : 'Dynamite' Hackathon : Fortitude Valley, Australia 21) 13 Jul, Fri-Sun : Blockchain Hackathon : Zürich, Switzerland 22) 13 Jul, Fri-Sat : Codefest Nigeria Hackathon (Abuja) : ABUJA, Nigeria 23) 13 Jul, Fri-Sun : Hack The Future: Between Earth & Sky : Choctaw, USA 24) 13 Jul, Fri-Sat : Pride Hacks/Axiona - Hackerfest : Montréal, Canada 25) 13 Jul, Fri-Sun : Code For Cognitive Aid : Portola Valley, United States
Flowchart:
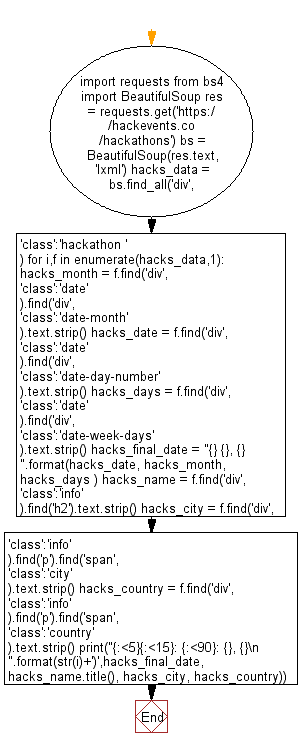
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find the live weather report (temperature, wind speed, description and weather) of a given city.
Next: Write a Python program to download IMDB's Top 250 data (movie name, Initial release, director name and stars).
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics