Pandas SQL Query: Calculate minimum, maximum and mean salary from employees file
22. Calculate Minimum, Maximum, and Mean Salary
Write a Pandas program to calculate minimum, maximum and mean salary from employees file.
EMPLOYEES.csv
Sample Solution :
Python Code :
import pandas as pd
employees = pd.read_csv(r"EMPLOYEES.csv")
departments = pd.read_csv(r"DEPARTMENTS.csv")
job_history = pd.read_csv(r"JOB_HISTORY.csv")
jobs = pd.read_csv(r"JOBS.csv")
countries = pd.read_csv(r"COUNTRIES.csv")
regions = pd.read_csv(r"REGIONS.csv")
locations = pd.read_csv(r"LOCATIONS.csv")
result = job_history.groupby(['employee_id'])
print(employees.agg({'salary': ['min', 'max', 'mean', 'median']}))
Sample Output:
salary min 2100.000000 max 24000.000000 mean 6461.682243 median 6200.000000
Equivalent SQL Syntax:
SELECT * FROM jobs ORDER BY job_title;
Click to view the table contain:
For more Practice: Solve these Related Problems:
- Write a Pandas program to calculate and display the minimum, maximum, and mean salary from EMPLOYEES.csv using aggregate functions.
- Write a Pandas program to compute these salary statistics grouped by department and display the results.
- Write a Pandas program to calculate salary statistics after filtering out extreme outliers from EMPLOYEES.csv.
- Write a Pandas program to compute and display the salary minimum, maximum, and mean, and then plot these statistics using a bar chart.
Go to:
Previous: Write a Pandas program to display the ID for those employees who did two or more jobs in the past.
Next: Write a Pandas program to display the details of jobs in descending sequence on job title.
Python Code Editor:
Structure of HR database :
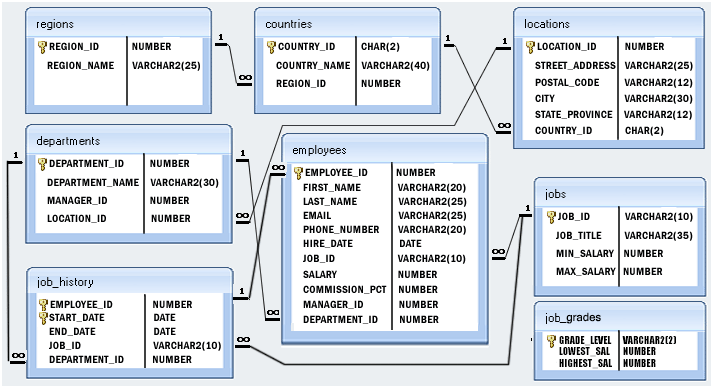
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?