Python Exercises, Practice, Solution
What is Python language?
Python is a widely used high-level, general-purpose, interpreted, dynamic programming language. Its design philosophy emphasizes code readability, and its syntax allows programmers to express concepts in fewer lines of code than possible in languages such as C++ or Java.
Python supports multiple programming paradigms, including object-oriented, imperative and functional programming or procedural styles. It features a dynamic type system and automatic memory management and has a large and comprehensive standard library
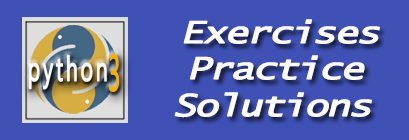
The best way we learn anything is by practice and exercise questions. We have started this section for those (beginner to intermediate) who are familiar with Python.
Hope, these exercises help you to improve your Python coding skills. Currently, following sections are available, we are working hard to add more exercises .... Happy Coding!
You may read our Python tutorial before solving the following exercises.
Latest Articles : Python Interview Questions and Answers Python PyQt
List of Python Exercises :
Python Basics- Python Basic (Part -I) [ 150 Exercises with Solution ]
- Python Basic (Part -II) [ 150 Exercises with Solution ]
- Python Programming Puzzles [ 100 Exercises with Solution ]
- Mastering Python [ 150 Exercises with Solution ]
- Python Conditional statements and loops [ 44 Exercises with Solution]
- Recursion [ 11 Exercises with Solution ]
- Python Data Types - String [ 113 Exercises with Solution ]
- Python JSON [ 9 Exercises with Solution ]
- Python Data Types - List [ 281 Exercises with Solution ]
- Python Data Types - List Advanced [ 15 Exercises with Solution ]
- Python Data Types - Dictionary [ 80 Exercises with Solution ]
- Python Data Types - Tuple [ 33 Exercises with Solution ]
- Python Data Types - Sets [ 30 Exercises with Solution ]
- Python Data Types - Collections [ 36 Exercises with Solution ]
- Python Array [ 24 Exercises with Solution ]
- Python Enum [ 5 Exercises with Solution ]
- Python Unit test [ 10 Exercises with Solution ]
- Python Exception Handling [ 10 exercises with solution ]
- Python Object-Oriented Programming [ 11 Exercises with Solution ]
- Python Decorator [ 12 Exercises with Solution ]
- Python functions [ 21 Exercises with Solution ]
- Python Lambda [ 52 Exercises with Solution ]
- Python Map [ 17 Exercises with Solution ]
- Python Itertools [ 44 exercises with solution ]
- Python - Filter Function [ 11 Exercises with Solution ]
- Python Date Time [ 63 Exercises with Solution ]
- Python Pendulum (DATETIMES made easy) Module [34 Exercises with Solution]
- Python File Input Output [ 21 Exercises with Solution ]
- Python CSV File Reading and Writing [ 11 exercises with solution ]
- Search and Sorting [ 39 Exercises with Solution ]
- Linked List [ 14 Exercises with Solution ]
- Binary Search Tree [ 6 Exercises with Solution ]
- Python heap queue algorithm [ 29 exercises with solution ]
- Python Bisect [ 9 Exercises with Solution ]
- Python Boolean Data Type [ 10 Exercises with Solution ]
- Python None Data Type [ 10 Exercises with Solution ]
- Python Bytes and Byte Arrays Data Type [ 10 Exercises with Solution ]
- Python Memory Views Data Type [ 10 Exercises with Solution ]
- Python frozenset Views [ 10 Exercises with Solution ]
- Python NamedTuple [ 9 Exercises with Solution ]
- Python OrderedDict [ 10 Exercises with Solution ]
- Python Counter [ 10 Exercises with Solution ]
- Python Ellipsis (...) [ 9 Exercises with Solution ]
- Python Multi-threading and Concurrency [ 7 exercises with solution ]
- Python Asynchronous [ 8 Exercises with Solution ]
- Python built-in Modules [ 31 Exercises with Solution ]
- Python Operating System Services [ 18 Exercises with Solution ]
- Python Math [ 94 Exercises with Solution ]
- Python Requests [ 9 exercises with solution ]
- Python SQLite Database [ 13 Exercises with Solution ]
- Python SQLAlchemy [ 14 exercises with solution ]
- Python - PPrint [ 6 Exercises with Solution ]
- Python - Cyber Security [ 10 Exercises with Solution ]
- Python Generators Yield [ 17 exercises with solution ]
- More to come
Python GUI Tkinter, PyQt
- Python Tkinter Home
- Python Tkinter Basic [ 16 Exercises with Solution ]
- Python Tkinter layout management [ 11 Exercises with Solution ]
- Python Tkinter widgets [ 22 Exercises with Solution ]
- Python Tkinter Dialogs and File Handling [ 13 Exercises with Solution ]
- Python Tkinter Canvas and Graphics [ 14 Exercises with Solution ]
- Python Tkinter Events and Event Handling [ 13 Exercises with Solution ]
- Python Tkinter Customs Widgets and Themes [ 12 Exercises with Solution ]
- Python Tkinter - File Operations and Integration [12 exercises with solution]
- Python PyQt Basic [10 exercises with solution]
- Python PyQt Widgets[12 exercises with solution]
- Python PyQt Connecting Signals to Slots [15 exercises with solution]
- Python PyQt Event Handling [10 exercises with solution]
Python Challenges :
- Python Challenges: Part -1 [ 1- 64 ]
- More to come
Python Projects :
- Python Numbers : [ 11 Mini Projects with solution ]
- Python Web Programming: [ 12 Mini Projects with solution ]
- 100 Python Projects for Beginners with solution.
- Python Projects: Novel Coronavirus (COVID-19) [ 14 Exercises with Solution ]
- More to come
Learn Python packages using Exercises, Practice, Solution and explanation
Python urllib3 :
Python Metaprogramming :
Python GeoPy Package :
Python BeautifulSoup :
Python Arrow Module :
Python Web Scraping :
Python Natural Language Toolkit :
Python NumPy :
- Python NumPy Basic [ 59 Exercises with Solution ]
- Python NumPy arrays [ 205 Exercises with Solution ]
- Python NumPy Mathematics [ 41 Exercises with Solution ]
- Python NumPy Linear Algebra [ 19 Exercises with Solution ]
- Python NumPy Statistics [ 14 Exercises with Solution ]
- Python NumPy Random [ 17 Exercises with Solution ]
- Python NumPy Sorting and Searching [ 9 Exercises with Solution ]
- NumPy Advanced Indexing [ 20 exercises with solution ]
- Python NumPy DateTime [ 7 Exercises with Solution ]
- Python NumPy String [ 22 Exercises with Solution ]
- NumPy Broadcasting [ 20 exercises with solution ]
- NumPy Memory Layout [ 19 exercises with solution ]
- NumPy Performance Optimization [ 20 exercises with solution ]
- NumPy Interoperability [ 20 exercises with solution ]
- NumPy I/O Operations [ 20 exercises with solution ]
- NumPy Universal Functions [ 20 exercises with solution ]
- NumPy Masked Arrays [ 20 exercises with solution ]
- NumPy Structured Arrays [ 20 exercises with solution ]
- NumPy Integration with SciPy [ 19 exercises with solution ]
- Advanced NumPy [ 33 exercises with solution ]
- Mastering NumPy [ 100 Exercises with Solutions ]
- More to come
Basic Operations and Arrays
Mathematics, Linear Algebra, and Statistics
Random Numbers
Sorting, Searching, and Indexing
Datetime and String Operations
Broadcasting and Memory Layout
Performance Optimization and Interoperability
Input/Output (I/O) Operations
Functions and Masked Arrays
Structured Arrays and SciPy Integration
Advanced Topics and Mastery
Python Pandas :
- Python Pandas Home
- Pandas Data Series [ 40 exercises with solution ]
- Pandas DataFrame [ 81 exercises with solution ]
- Pandas Index [ 26 exercises with solution ]
- Pandas String and Regular Expression [ 41 exercises with solution ]
- Pandas Joining and merging DataFrame [ 15 exercises with solution ]
- Pandas Grouping and Aggregating [ 32 exercises with solution ]
- Pandas Time Series [ 32 exercises with solution ]
- Pandas Filter [ 27 exercises with solution ]
- Pandas GroupBy [ 32 exercises with solution ]
- Pandas Handling Missing Values [ 20 exercises with solution ]
- Pandas Style [ 15 exercises with solution ]
- Pandas Excel Data Analysis [ 25 exercises with solution ]
- Pandas Pivot Table [ 32 exercises with solution ]
- Pandas Datetime [ 25 exercises with solution ]
- Pandas Plotting [ 19 exercises with solution ]
- Pandas Performance Optimization [ 20 exercises with solution ]
- Pandas Advanced Indexing and Slicing [ 15 exercises with solution ]
- Pandas SQL database Queries [ 24 exercises with solution ]
- Pandas Resampling and Frequency Conversion [ 15 exercises with solution ]
- Pandas Advanced Grouping and Aggregation [ 15 exercises with solution ]
- Pandas IMDb Movies Queries [ 17 exercises with solution ]
- Mastering NumPy: 100 Exercises with solutions for Python numerical computing
- Pandas Practice Set-1 [ 65 exercises with solution ]
- More to come
Pandas and NumPy Exercises :
- Pandas and NumPy for Data Analysis [ 37 Exercises ]
- More to come
Python Machine Learning :
More...
Note : Download Python from https://www.python.org/ftp/python/3.2/ and install in your system to execute the Python programs. You can read our Python Installation on Fedora Linux and Windows 7, if you are unfamiliar to Python installation.
You may accomplish the same task (solution of the exercises) in various ways, therefore the ways described here are not the only ways to do stuff. Rather, it would be great, if this helps you anyway to choose your own methods.
List of Exercises with Solutions :
- HTML CSS Exercises, Practice, Solution
- JavaScript Exercises, Practice, Solution
- jQuery Exercises, Practice, Solution
- jQuery-UI Exercises, Practice, Solution
- CoffeeScript Exercises, Practice, Solution
- Twitter Bootstrap Exercises, Practice, Solution
- C Programming Exercises, Practice, Solution
- C# Sharp Programming Exercises, Practice, Solution
- PHP Exercises, Practice, Solution
- Python Exercises, Practice, Solution
- R Programming Exercises, Practice, Solution
- Java Exercises, Practice, Solution
- SQL Exercises, Practice, Solution
- MySQL Exercises, Practice, Solution
- PostgreSQL Exercises, Practice, Solution
- SQLite Exercises, Practice, Solution
- MongoDB Exercises, Practice, Solution
More to Come !
Do not submit any solution of the above exercises at here, if you want to contribute go to the appropriate exercise page.
[ Want to contribute to Python exercises? Send your code (attached with a .zip file) to us at w3resource[at]yahoo[dot]com. Please avoid copyrighted materials.]
Test your Python skills with w3resource's quiz
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics