Python: iter() function
iter() function
The iter() function returns an iterator object.
Version:
(Python 3.2.5)
Syntax:
iter(object[, sentinel])
Parameter:
Name | Description | Required / Optional |
---|---|---|
object | An object whose iterator has to be created (can be sets, tuples, etc.) | Required |
sentinel | special value that is used to represent the end of a sequence | Optional |
The following example reads a file until the readline() method returns an empty string:
with open('mydata.txt') as fp: for line in iter(fp.readline, ''): process_line(line)
Example: Python: iter() function
# list of letters
letters = ['P', 'y', 't', 'h', 'o', 'n']
lettersIter = iter(letters)
# prints 'P'
print(next(lettersIter))
# prints 'y'
print(next(lettersIter))
# prints 't'
print(next(lettersIter))
# prints 'h'
print(next(lettersIter))
# prints 'o'
print(next(lettersIter))
# prints 'n'
print(next(lettersIter))
Output:
P y t h o n
Pictorial Presentation:
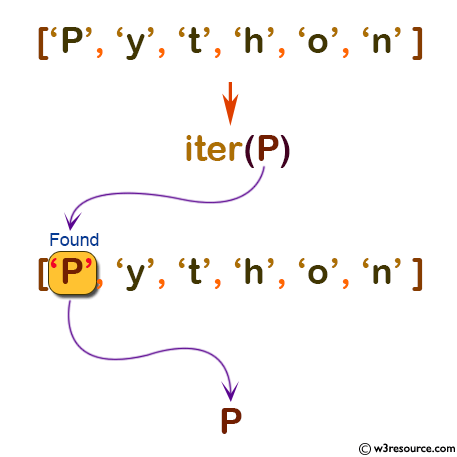
Pictorial Presentation:
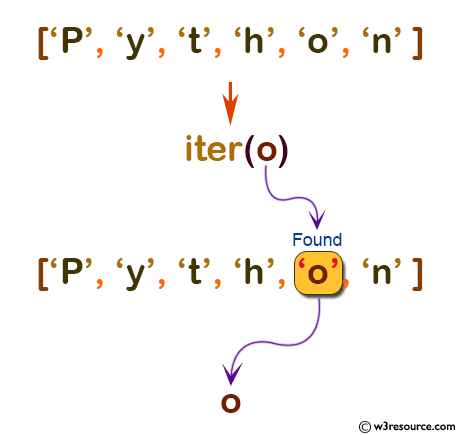
Python Code Editor:
Previous: issubclass()
Next: len()
Test your Python skills with w3resource's quiz
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python/built-in-function/iter.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics