Python: list() function
list() function
The list() function is used to create a list object (list is actually a mutable sequence type).
Read more about list from Python List and Python Exercises.
Version
(Python 3.2.5)
Syntax:
list([iterable])
Parameter:
Name | Description | Required / Optional |
---|---|---|
iterable | A sequence, collection or an iterator object. | Optional |
Example: Python list() function
# Example list
ExampleList = ['P', 'y', 't', 'h', 'o', 'n']
print(list(ExampleList))
Output:
['P', 'y', 't', 'h', 'o', 'n']
Pictorial Presentation:
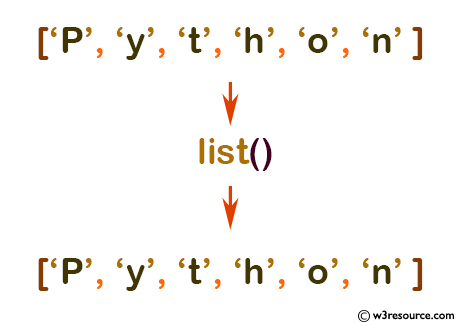
Example: Python list() function with iterable
class PythonList:
def __init__(x, max):
x.max = max
def __iter__(x):
x.number = 0
return x
def __next__(x):
if(x.number >= x.max):
raise StopIteration
result = 3 ** x.number
x.number += 1
return result
PythonList = PythonList(7)
PythonListIter = iter(PythonList)
print(list(PythonListIter))
Output:
[1, 3, 9, 27, 81, 243, 729]
Python Code Editor:
Previous: len()
Next: locals()
Test your Python skills with w3resource's quiz