Python List index() Method
index() Method
The index method() is used to get the zero-based index in the list of the first item whose value is given.
The index() method is a useful tool for locating the position of elements in a list. It offers flexibility with optional start and end parameters to narrow the search to a specific subsequence of the list. Understanding how to handle exceptions like ValueError is essential for robust code that gracefully manages the absence of the searched element.
Visual Explanation:
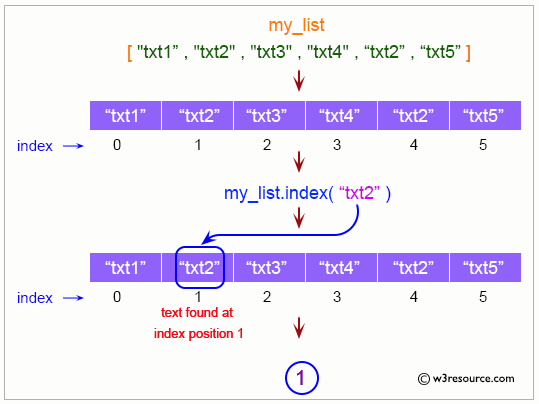
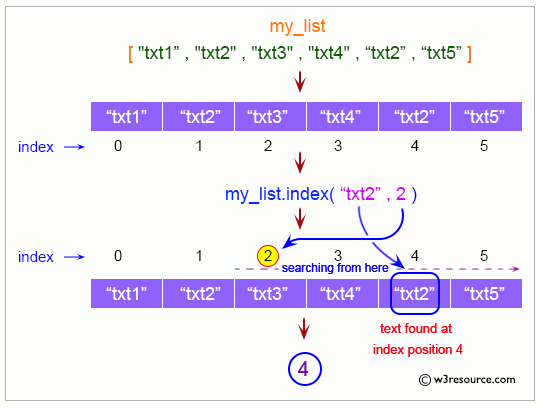
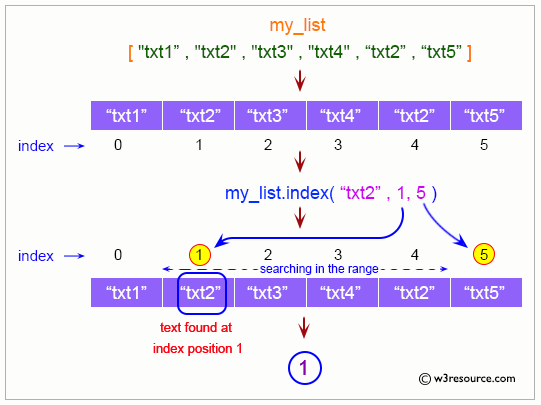
Syntax:
list.index(element, start, end)
Parameters:
- element: The element to be searched.
- start (optional): The position to start the search.
- end (optional): The position to end the search.
Return Value: The zero-based index of the first occurrence of the specified element.
Error Handling: Raises a ValueError if the element is not found.
Example 1: Find the Index of an Element
# colors list
colors = ['Red', 'Green', 'Black', 'Green']
print("Original list:")
print(colors)
# Find the index of the first occurrence of 'Green'
index_green = colors.index('Green')
print("Position at the first occurrence of 'Green':")
print(index_green)
Output:
Original list: ['Red', 'Green', 'Black', 'Green'] Position at the first occurrence of 'Green': 1
Example 2: Find the Index of an Element After a Given Position
# fruits list
fruits = ['orange', 'apple', 'pear', 'banana', 'kiwi', 'apple', 'banana']
print("Original list:")
print(fruits)
# Find the index of 'apple' starting the search from position 4
index_apple = fruits.index('apple', 4)
print("Position of 'apple' after the specified position:")
print(index_apple)
Output:
Original list: ['orange', 'apple', 'pear', 'banana', 'kiwi', 'apple', 'banana'] Position of 'apple' after the specified position: 5
Example 3: Find the Index of an Element Within a Specific Range
# fruits list
fruits = ['orange', 'apple', 'pear', 'banana', 'kiwi', 'apple', 'banana']
print("Original list:")
print(fruits)
# Find the index of 'apple' within the range of index 2 to 6
index_apple_range = fruits.index('apple', 2, 6)
print("Position of 'apple' within the specified range:")
print(index_apple_range)
Output:
Original list: ['orange', 'apple', 'pear', 'banana', 'kiwi', 'apple', 'banana'] Position of 'apple' within the specified range: 5
Example 4: Handling ValueError
#Attempting to find an element that does not exist within the specified range raises a ValueError.
#fruits list
fruits = ['orange', 'apple', 'pear', 'banana', 'kiwi', 'apple', 'banana']
print("Original list:")
print(fruits)
# Attempt to find 'apple' in a range where it does not exist
try:
index_nonexistent = fruits.index('apple', 2, 3)
print("Position of 'apple' within the specified range:")
print(index_nonexistent)
except ValueError as e:
print("Error:", e)
Output:
Original list: ['orange', 'apple', 'pear', 'banana', 'kiwi', 'apple', 'banana'] Error: 'apple' is not in list
Python list index() Method FAQ
1. What does the Python list index() method do?
The index() method returns the zero-based index of the first occurrence of a specified element in a list.
2. Can the Python list index() method search for an element within a specific range?
Yes, the index() method can search within a specific range if optional start and end parameters are provided.
3. What happens if the element is not found in the list?
The index() method raises a ValueError if the specified element is not found in the list.
4. Are the start and end parameters mandatory?
No, the start and end parameters are optional. If not provided, the search will cover the entire list.
5. Is the Python list index() method case-sensitive when searching for string elements?
Yes, the index() method is case-sensitive when searching for string elements.
6. Can the Python list index() method be used with any data type?
Yes, the index() method can be used to search for elements of any data type, including strings, numbers, lists, and tuples.
7. Does the Python list index() method modify the original list?
No, the index() method does not modify the original list. It only returns the index of the specified element.
8. What is the return value of the Python list index() method?
The index() method returns the zero-based index of the first occurrence of the specified element.
9. Can the Python list index() method handle nested lists?
The index() method can search for nested lists as elements, but it does not search within nested lists.
10. How does the Python list index() method behave if multiple occurrences of the element exist?
The index() method returns the index of the first occurrence of the specified element.
Python Code Editor:
Previous:Python List extend() Method
Next: Python List insert() Method
Test your Python skills with w3resource's quiz
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics