Python List extend() Method
extend() Method
The extend() method is used to extend a list by appending all the items from the iterable.
The extend() method is a powerful and flexible way to add multiple elements from an iterable to the end of a list. It allows for easy combination of lists and other iterables, making it a valuable tool for managing collections of data in Python.
Visual Explanation:
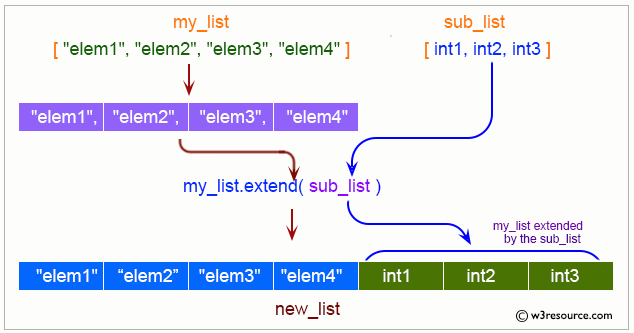
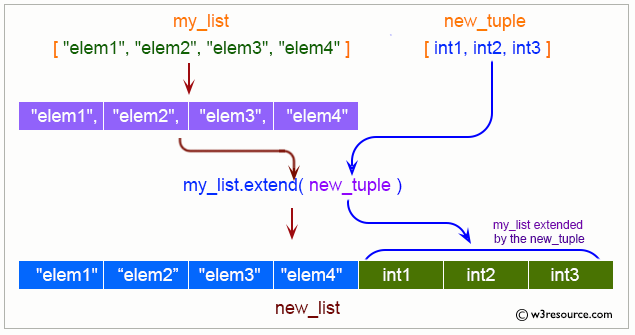
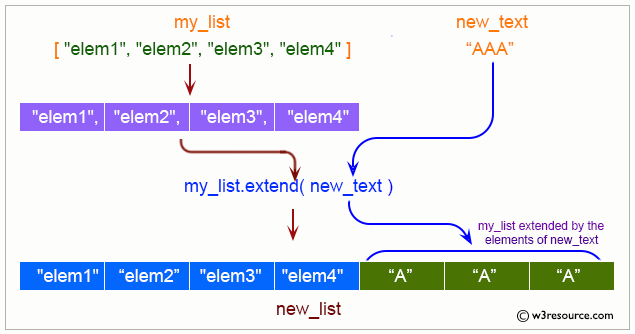
Syntax:
list1.extend(iterable)
Parameter:
- iterable: An iterable (e.g., list, tuple, set, string) whose elements will be added to the list.
Return Value: The extend() method does not return any value. It modifies the original list.
Equivalent Command
The extend() method is equivalent to:
a[len(a):] = iterable
Example 1: Extending a List with Another List
# First list
color1 = ['Red', 'Green', 'Black']
print("First list:")
print(color1)
# Second list
color2 = ['White', 'Orange']
print("Second list:")
print(color2)
# Extend the first list with the second list
color1.extend(color2)
print("List after extending:")
print(color1)
Output:
First list: ['Red', 'Green', 'Black'] Second list: ['White', 'Orange'] List after extending: ['Red', 'Green', 'Black', 'White', 'Orange']
Example 2: Adding Elements of a Tuple to a List
# Original list
colors = ['Red', 'Green', 'Black']
print("Original list:")
print(colors)
# Tuple to add
nums = (1, 2, 3, 4, 5)
print("Tuple to add:")
print(nums)
# Extend the list with elements from the tuple
colors.extend(nums)
print("List after extending:")
print(colors)
Output:
Original list: ['Red', 'Green', 'Black'] Tuple to add: (1, 2, 3, 4, 5) List after extending: ['Red', 'Green', 'Black', 1, 2, 3, 4, 5]
Example 3: Adding Characters of a String to a List
# Original list
colors = ['Red', 'Green', 'Black']
print("Original list:")
print(colors)
# String to add
text = "Pink"
print("String to add:")
print(text)
# Extend the list with characters from the string
colors.extend(text)
print("List after extending:")
print(colors)
Output:
Original list: ['Red', 'Green', 'Black'] String to add: Pink List after extending: ['Red', 'Green', 'Black', 'P', 'i', 'n', 'k']
Example 4: Extending a List with a Set
# Original list
numbers = [1, 2, 3]
print("Original list:")
print(numbers)
# Set to add
num_set = {4, 5, 6}
print("Set to add:")
print(num_set)
# Extend the list with elements from the set
numbers.extend(num_set)
print("List after extending:")
print(numbers)
Output:
Original list: [1, 2, 3] Set to add: {4, 5, 6} List after extending: [1, 2, 3, 4, 5, 6]
Python list extend() Method FAQ
1. What does the Python list extend() method do?
The Python list extend() method adds all the elements of an iterable to the end of the list.
2. What types of iterables can be used with the extend() method?
Any iterable, such as lists, tuples, sets, or strings, can be used with the extend() method.
3. Does the Python list extend() method modify the original list?
Yes, the Python list extend() method modifies the original list in place.
4. Does the Python list extend() method return any value?
No, the Python list extend() method does not return a value. It only modifies the list.
5. Can the Python list extend() method be used to concatenate two lists?
Yes, the Python list extend() method can concatenate another list to the end of the original list.
6. How is Python list extend() different from append()?
The append() method adds its argument as a single element to the end of the list, while extend() adds each element of the iterable to the list.
7. Is there a performance difference between Python list extend() and using a loop to add elements?
The extend() method is generally more efficient than using a loop to add elements individually.
8. Can Python list extend() be used with custom iterable objects?
Yes, as long as the custom object implements the iterable protocol, it can be used with extend().
9. What happens if you pass a non-iterable to the Python list extend() method?
Passing a non-iterable to the extend() method will result in a TypeError.
10. Is it possible to use Python list extend() with an empty iterable?
Yes, using extend() with an empty iterable will have no effect on the original list.
Python Code Editor:
Previous: Python List count() Method.
Next: Python List index() Method.
Test your Python skills with w3resource's quiz