Python List insert() Method
insert() Method
The insert() method is used to insert an item at a given position in a list.
The insert() method is a powerful tool for inserting elements into a list at a specific position. It allows for precise control over the placement of new elements, whether you're adding single items or entire lists. Understanding how to use this method effectively can help you manage and manipulate lists with greater flexibility.
Visual Explanation:
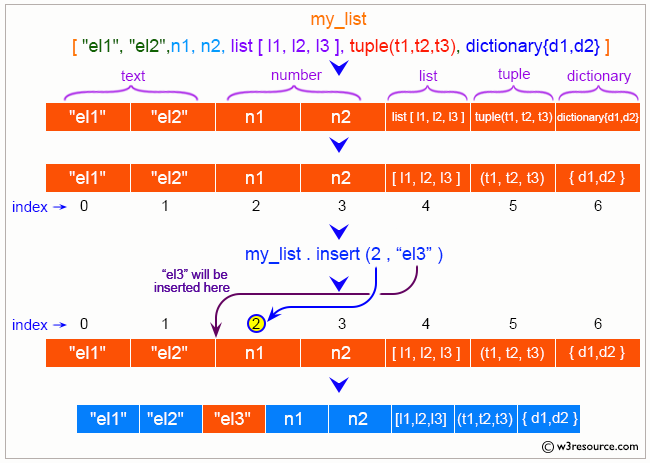
So a.insert(0, x) inserts at the front of the list, and a.insert(len(a), x) is equivalent to a.append(x).
Syntax:
list.insert(i, element)
Parameter:
- index - The first argument is the index of the element before which to insert.
- element - Element to be inserted in the list.
Return Value from insert() method
None. It only updates the current list.
Example 1: Inserting an Element at a Specific Position
# colors list
colors = ['Red', 'Green', 'Black']
print("Original list:")
print(colors)
# Insert 'White' at the second position (index 1)
colors.insert(1, 'White')
print("List after inserting 'White' at the second position:")
print(colors)
Output:
Original list: ['Red', 'Green', 'Black'] List after inserting 'White' at the second position: ['Red', 'White', 'Green', 'Black']
Example 2: Inserting a List into a List of Lists
# List of lists
nums = [[1, 2, 3], [1, 2], [4, 5, 6], [4, 5]]
print("Original list of lists:")
print(nums)
# Insert a new list at the third position (index 2)
new_list = [-1, 0, 2]
nums.insert(2, new_list)
print("List after inserting a new list at the third position:")
print(nums)
Output:
Original list of lists: [[1, 2, 3], [1, 2], [4, 5, 6], [4, 5]] List after inserting a new list at the third position: [[1, 2, 3], [1, 2], [-1, 0, 2], [4, 5, 6], [4, 5]]
Example 3: Inserting Elements at the Beginning and End
# numbers list
numbers = [2, 3, 4]
print("Original list:")
print(numbers)
# Insert at the beginning
numbers.insert(0, 1)
print("List after inserting 1 at the beginning:")
print(numbers)
# Insert at the end
numbers.insert(len(numbers), 5)
print("List after inserting 5 at the end:")
print(numbers)
Output:
Original list: [2, 3, 4] List after inserting 1 at the beginning: [1, 2, 3, 4] List after inserting 5 at the end: [1, 2, 3, 4, 5]
Example 4: Inserting an Element into a List of Strings
# list of fruits
fruits = ['apple', 'banana', 'cherry']
print("Original list:")
print(fruits)
# Insert 'orange' at the second position
fruits.insert(1, 'orange')
print("List after inserting 'orange' at the second position:")
print(fruits)
Output:
Original list: ['apple', 'banana', 'cherry'] List after inserting 'orange' at the second position: ['apple', 'orange', 'banana', 'cherry']
Python list insert() Method FAQ
1. What does Python list insert() method do?
The insert() method inserts an element at a specified position in a list.
2. Does the Python list insert() method modify the original list?
Yes, the insert() method modifies the original list in place.
3. Can the Python list insert() method add an element at the beginning of the list?
Yes, by specifying the index as 0, the insert() method can add an element at the beginning of the list.
4. Can the Python list insert() method add an element at the end of the list?
Yes, by specifying the index as the length of the list, the insert() method can add an element at the end of the list.
5. What happens if the specified Python list index is out of range?
If the index is greater than the length of the list, the element is inserted at the end of the list. If the index is negative, it is counted from the end of the list.
6. Can the Python list insert() method be used to add multiple elements at once?
No, the insert() method can only insert one element at a specified position. To add multiple elements, you need to call insert() multiple times or use another method like extend().
7. What types of elements can be inserted using the Python list insert() method?
Any type of element (e.g., numbers, strings, lists, tuples) can be inserted into a list using the insert() method.
8. Does the Python list insert() method return any value?
No, the insert() method does not return any value. It only updates the list.
9. Can the Python list insert() method be used with lists that contain nested lists?
Yes, the insert() method can be used to insert elements, including nested lists, at any specified position in a list.
10. How does the Python list insert() method handle duplicate elements?
The Python list insert() method does not check for duplicates. It inserts the specified element at the given position, regardless of whether the element already exists in the list.
Python Code Editor:
Previous: Python List index() Method.
Next: Python List pop() Method.
Test your Python skills with w3resource's quiz
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics