Python List count() Method
count() Method
The count() method is used to get the number of times a specified element appears in a given list.
The count() method is a versatile and simple tool for counting the occurrences of elements in a list. Whether we are working with simple data types like strings and numbers or more complex types like lists, tuples, and custom objects, the count() method can help you quickly determine how many times an element appears in your list.
Visual Explanation:
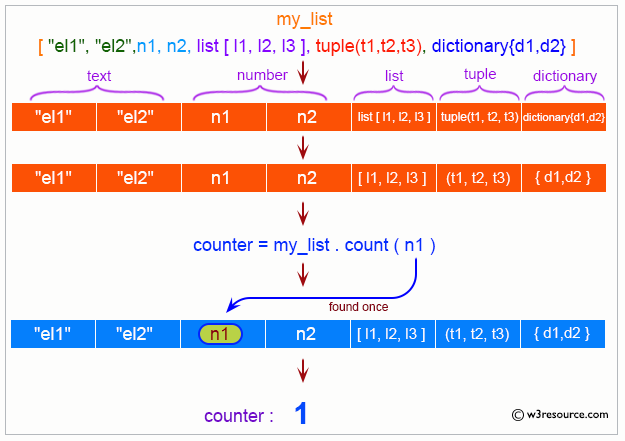
Syntax:
list.count(element)
Parameter:
- element - Required. The element is to be counted.. Type -> any type (string, number, list, tuple, etc.).
Return value from count() method
The number of times an element appears in the list is returned.
Example 1: Counting Occurrences of a String
# colors list
colors = ['Red', 'Green', 'Black', 'Red', 'Green']
print("Original list:")
print(colors)
# Count occurrences of 'Red'
red_count = colors.count('Red')
print("Number of times 'Red' appears in the colors list:")
print(red_count)
Output:
Original list: ['Red', 'Green', 'Black', 'Red', 'Green'] Number of times 'Red' appears in the colors list: 2
Example 2: Counting Occurrences of a Tuple and a List within a List
# List containing other lists and tuples
mixed_items = [[1, 2], (1, 2, 3), [3, 4, 5], [1, 2], (1, 2, 3)]
print("Original list:")
print(mixed_items)
# Count occurrences of the list [1, 2]
list_count = mixed_items.count([1, 2])
print("Number of times the list [1, 2] appears in the list:")
print(list_count)
# Count occurrences of the tuple (1, 2, 3)
tuple_count = mixed_items.count((1, 2, 3))
print("Number of times the tuple (1, 2, 3) appears in the list:")
print(tuple_count)
Output:
Original list: [[1, 2], (1, 2, 3), [3, 4, 5], [1, 2], (1, 2, 3)] Number of times the list [1, 2] appears in the list: 2 Number of times the tuple (1, 2, 3) appears in the list: 2
Example 3: Counting Occurrences of Integers
# numbers list
numbers = [1, 2, 3, 4, 5, 3, 3, 2, 1]
print("Original list:")
print(numbers)
# Count occurrences of the number 3
three_count = numbers.count(3)
print("Number of times the number 3 appears in the numbers list:")
print(three_count)
Output:
Original list: [1, 2, 3, 4, 5, 3, 3, 2, 1] Number of times the number 3 appears in the numbers list: 3
Example 4: Counting Occurrences of Custom Objects
# Define a custom class
class CustomObject:
def __init__(self, value):
self.value = value
def __eq__(self, other):
return self.value == other.value
# Create a list of custom objects
custom_list = [CustomObject(1), CustomObject(2), CustomObject(1), CustomObject(3)]
print("Original list of custom objects:")
# Count occurrences of a specific custom object
custom_obj = CustomObject(1)
custom_count = custom_list.count(custom_obj)
print("Number of times CustomObject(1) appears in the custom list:")
print(custom_count)
Output:
Original list of custom objects: Number of times CustomObject(1) appears in the custom list: 2
Python list count() Method FAQ
Python count() Method FAQ
1. What does the Python list count() method do?
The count() method returns the number of times a specified element appears in a list.
2. Can Python list count() be used with different data types?
Yes, count() can be used with any data type, including strings, numbers, lists, tuples, and custom objects.
3. Does the Python list count() method modify the original list?
No, the count() method does not modify the original list. It only counts the occurrences of the specified element.
4. What is the return value of the Python list count() method?
The count() method returns an integer representing the number of times the specified element appears in the list.
5. Can Python list count() be used to count elements in nested lists?
Yes, count() can be used to count elements in nested lists, but it only counts exact matches of the specified element.
6. Is Python list count() case-sensitive when used with strings?
Yes, the count() method is case-sensitive when counting string elements in a list.
7. How does Python list count() handle custom objects?
The count() method uses the __eq__ method to compare custom objects. If the __eq__ method is defined, count() will use it to determine if objects are equal.
8. Does the Python list count() method require the element to exist in the list?
No, if the specified element does not exist in the list, the count() method returns 0.
9. Can the count() method be used on other data structures like tuples or sets?
No, the count() method is specific to lists. However, similar methods exist for other data structures.
10. Is there a performance consideration when using Python list count() on large lists?
Yes, the count() method performs a linear search, so its performance can be impacted by the size of the list. It has a time complexity of O(n), where n is the number of elements in the list.
Python Code Editor:
Previous: Python List copy() Method.
Next: Python List extend() Method.
Test your Python skills with w3resource's quiz