Python List copy() Method
copy() Method
The copy() method returns a copy of a given list.
The copy() method is a simple and effective way to duplicate lists in Python. It performs a shallow copy, which is sufficient for lists without nested mutable objects. For lists with nested objects, use the copy.deepcopy() method from the copy module to ensure a complete, independent copy.
Visual Explanation:
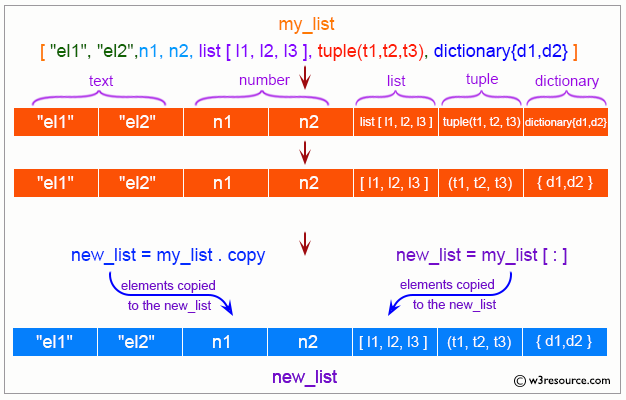
Syntax:
new_list = list.copy()
Parameter: No parameters
Return Value from clear() method
Returns a new list. It doesn't modify the original list.
Equivalent Command
You can achieve the same result using slicing:
new_list = original_list[:]
Example 1: Basic Usage of copy() Method
# Original list
colors = ['Red', 'Green', 'Black']
print("Original list:")
print(colors)
# Copy the list
new_colors = colors.copy()
print("Copied list:")
print(new_colors)
# Modify the new list
new_colors.append('White')
print("New list after adding an element:")
print(new_colors)
# Ensure the original list remains unchanged
print("Original list after modification of new list:")
print(colors)
Output:
Original list: ['Red', 'Green', 'Black'] Copied list: ['Red', 'Green', 'Black'] New list after adding an element: ['Red', 'Green', 'Black', 'White'] Original list after modification of new list: ['Red', 'Green', 'Black']
Example 2: Copying a List Using Slicing Syntax
# Original list
colors = ['Red', 'Green', 'Black']
print("Original list:")
print(colors)
# Copy the list using slicing
new_colors = colors[:]
print("Copied list using slicing:")
print(new_colors)
# Modify the new list
new_colors.append('White')
print("New list after adding an element:")
print(new_colors)
# Ensure the original list remains unchanged
print("Original list after modification of new list:")
print(colors)
Output:
Original list: ['Red', 'Green', 'Black'] Copied list using slicing: ['Red', 'Green', 'Black'] New list after adding an element: ['Red', 'Green', 'Black', 'White'] Original list after modification of new list: ['Red', 'Green', 'Black']
Example 3: Copying Nested Lists
It's important to note that copy() creates a shallow copy, meaning it only copies the references of nested lists.
# Original nested list
nested_list = [['Red', 'Green'], ['Black']]
print("Original nested list:")
print(nested_list)
# Copy the nested list
new_nested_list = nested_list.copy()
print("Copied nested list:")
print(new_nested_list)
# Modify the new nested list
new_nested_list[0].append('White')
print("New nested list after adding an element:")
print(new_nested_list)
# Check the original nested list
print("Original nested list after modification of new list:")
print(nested_list)
Output:
Original nested list: [['Red', 'Green'], ['Black']] Copied nested list: [['Red', 'Green'], ['Black']] New nested list after adding an element: [['Red', 'Green', 'White'], ['Black']] Original nested list after modification of new list: [['Red', 'Green', 'White'], ['Black']]
Example 4: Deep Copying a List
To create a deep copy of a list (which also copies nested lists), you need to use the copy module.
import copy
# Original nested list
nested_list = [['Red', 'Green'], ['Black']]
print("Original nested list:")
print(nested_list)
# Deep copy the nested list
deep_copied_list = copy.deepcopy(nested_list)
print("Deep copied nested list:")
print(deep_copied_list)
# Modify the deep copied list
deep_copied_list[0].append('White')
print("Deep copied list after adding an element:")
print(deep_copied_list)
# Ensure the original nested list remains unchanged
print("Original nested list after modification of deep copied list:")
print(nested_list)
Output:
Original nested list: [['Red', 'Green'], ['Black']] Deep copied nested list: [['Red', 'Green'], ['Black']] Deep copied list after adding an element: [['Red', 'Green', 'White'], ['Black']] Original nested list after modification of deep copied list: [['Red', 'Green'], ['Black']]
Python list copy() Method FAQ
1. What does the Python list copy() method do?
The copy() method creates a shallow copy of a list.
2. Does the Python list copy() method modify the original list?
No, the copy() method does not modify the original list. It returns a new list.
3. What type of copy does the Python list copy() method create?
The copy() method creates a shallow copy, meaning it only copies the references of nested objects.
4. Does the Python list copy() method take any parameters?
No, the copy() method does not take any parameters.
5. What is the return value of the Python list copy() method?
The copy() method returns a new list that is a shallow copy of the original list.
6. How is the Python list copy() method different from slicing for copying a list?
Functionally, the copy() method and slicing ([:]) produce the same result, both creating a shallow copy of the list.
7. Can the Python list copy() method be used with nested lists?
Yes, but it will only create a shallow copy, meaning nested lists will still reference the same objects as in the original list.
8. How do you create a deep copy of a list?
To create a deep copy, which duplicates nested objects as well, you should use the deepcopy() function from the copy module.
9. Is the Python list copy() method available for all Python list objects?
Yes, the copy() method is a built-in method available for all Python list objects.
10. Does the Python list copy() method work for other data structures like dictionaries or sets?
No, the copy() method is specific to lists. However, dictionaries and sets have their own copy() methods.
Python Code Editor:
Previous: Python List clear() Method.
Next: Python List count() Method.
Test your Python skills with w3resource's quiz
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics