Python List pop() Method
pop() Method
The pop() method is used to remove the item at the given position in a list, and return it. If no index is specified, a.pop() removes and returns the last item in the list.
The pop() method is a versatile and useful tool for list manipulation, allowing us to remove and retrieve elements from a list by specifying their index. It can handle both positive and negative indices, providing flexibility in accessing elements from different positions. Understanding the behavior of the pop() method is essential for effectively managing list data in Python.
Visual Explanation:
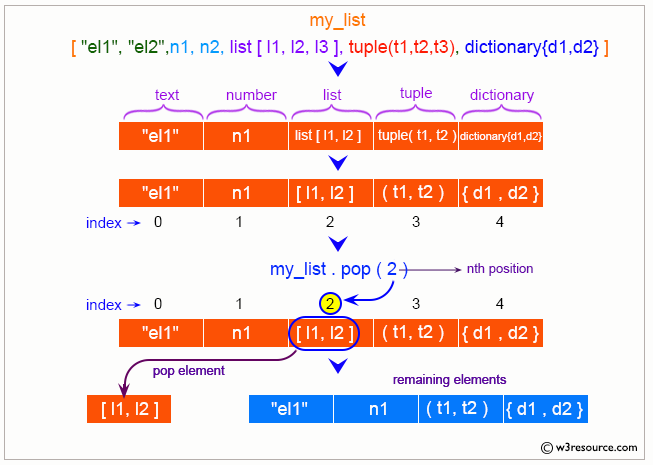
Syntax:
list.pop([i])
Parameters:
Optional. A number indicating the position of the element to be removed. Default value is -1, which returns the last item.
Return Value:
Return the removed element.
Example 1: Remove an Item at a Specified Index
# colors list
colors = ['Red', 'Green', 'Orange', 'Pink']
print("Original List:")
print(colors)
# Remove and return the 3rd item (index 2)
return_item = colors.pop(2)
print("Removed item:", return_item)
print("Updated List:", colors)
Output:
Original List: ['Red', 'Green', 'Orange', 'Pink'] Removed item: Orange Updated List: ['Red', 'Green', 'Pink']
Example 2: Remove and Return the Last Item
# colors list
colors = ['Red', 'Green', 'Orange', 'Pink']
print("Original List:")
print(colors)
# Remove and return the last item
return_item = colors.pop()
print("Removed item:", return_item)
print("Updated List:", colors)
Output:
Original List: ['Red', 'Green', 'Orange', 'Pink'] Removed item: Pink Updated List: ['Red', 'Green', 'Orange']
Example 3: Using Negative Indices
# colors list
colors = ['Red', 'Green', 'Orange', 'Pink']
print("Original List:")
print(colors)
# Remove and return the last item using -1
return_item_last = colors.pop(-1)
print("Removed last item:", return_item_last)
print("Updated List:", colors)
# Reset list
colors = ['Red', 'Green', 'Orange', 'Pink']
print("\nOriginal List:")
print(colors)
# Remove and return the second last item using -2
return_item_second_last = colors.pop(-2)
print("Removed second last item:", return_item_second_last)
print("Updated List:", colors)
Output:
Original List: ['Red', 'Green', 'Orange', 'Pink'] Removed last item: Pink Updated List: ['Red', 'Green', 'Orange'] Original List: ['Red', 'Green', 'Orange', 'Pink'] Removed second last item: Orange Updated List: ['Red', 'Green', 'Pink']
Example 4: Removing Elements from an Empty List
Attempting to remove an item from an empty list will raise an IndexError.
# empty list
empty_list = []
try:
empty_list.pop()
except IndexError as e:
print("Error:", e)
Output:
Error: pop from empty list
Python pop() Method FAQ
1. What does the Python list pop() method do?
The pop() method removes and returns an item from a list at a specified position.
2. What happens if no index is specified in Python list?
If no index is specified, the pop() method removes and returns the last item in the list.
3. Does the Python list pop() method modify the original list?
Yes, the pop() method modifies the original list by removing the specified element.
4. What is the return value of the Python list pop() method?
The pop() method returns the removed element.
5. Can the Python list pop() method be used with negative indices?
Yes, the pop() method can be used with negative indices to remove elements from the end of the list.
6. What happens if the specified index is out of range in a Python list?
If the specified index is out of range, the pop() method raises an IndexError.
7. Can the Python list pop() method be used to remove items from an empty list?
No, attempting to use pop() on an empty list will raise an IndexError.
8. Is the Python list pop() method exclusive to lists?
Yes, the pop() method is specific to lists. Other data structures may have similar methods with different behaviors.
9. How does the Python list pop() method handle multiple occurrences of the same element?
The pop() method removes the element at the specified index, regardless of whether the element appears multiple times in the list.
10. Does the Python list pop() method require the element to exist in the list?
No, the pop() method operates based on the index, not the element itself. The specified index must exist within the list's range.
Python Code Editor:
Previous: Python List insert() Method.
Next: Python List remove() Method.
Test your Python skills with w3resource's quiz
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics