Python List remove() Method
remove() Method
The remove() method is used to remove the first occurrence of a given element from a list.
The remove() method is a useful tool for list manipulation in Python. It allows you to remove the first occurrence of a specified element from a list. However, it is important to handle potential ValueError exceptions when the specified element does not exist in the list.
Visual Explanation:
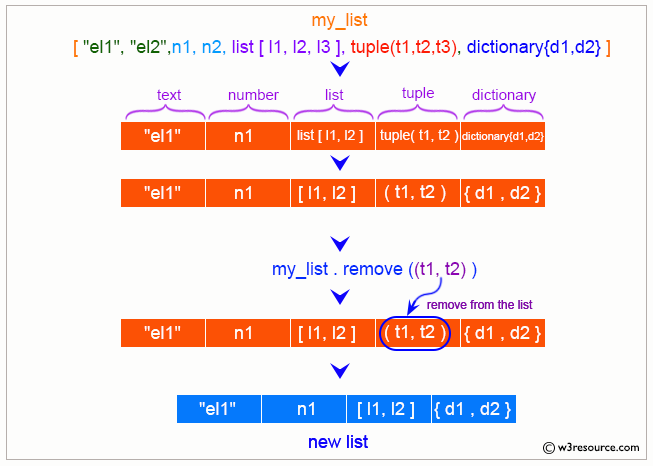
Syntax:
list.remove(x)
Parameter:
x: The element to be removed. This can be of any type (e.g., string, number, list).
Return Value: The remove() method does not return any value. It updates the list in place.
Error Handling: Raises a ValueError if the specified element is not found in the list.
Example 1: Remove an Element from a List
# colors list
colors = ['Red', 'Green', 'Orange', 'Pink']
print("Original List:")
print(colors)
# Remove 'Green' from the list
colors.remove('Green')
print("List after removing 'Green':")
print(colors)
Output:
Original List: ['Red', 'Green', 'Orange', 'Pink'] List after removing 'Green': ['Red', 'Orange', 'Pink']
Example 2: Handling Removal of a Non-Existent Element
# colors list
colors = ['Red', 'Green', 'Orange', 'Pink']
print("Original List:")
print(colors)
# Attempt to remove 'White' from the list
try:
colors.remove('White')
except ValueError as e:
print("Error:", e)
Output:
Original List: ['Red', 'Green', 'Orange', 'Pink'] Error: list.remove(x): x not in list
Example 3: Removing an Element from a List of Numbers
# numbers list
numbers = [1, 2, 3, 4, 3, 5]
print("Original List:")
print(numbers)
# Remove the first occurrence of 3
numbers.remove(3)
print("List after removing the first occurrence of 3:")
print(numbers)
Output:
Original List: [1, 2, 3, 4, 3, 5] List after removing the first occurrence of 3: [1, 2, 4, 3, 5]
Example 4: Removing an Element from a List of Lists
# list of lists
nested_list = [[1, 2], [3, 4], [1, 2], [5, 6]]
print("Original List:")
print(nested_list)
# Remove the first occurrence of [1, 2]
nested_list.remove([1, 2])
print("List after removing the first occurrence of [1, 2]:")
print(nested_list)
Output:
Original List: [[1, 2], [3, 4], [1, 2], [5, 6]] List after removing the first occurrence of [1, 2]: [[3, 4], [1, 2], [5, 6]]
Python list remove() Method FAQ
1. What does the Python list remove() method do?
The remove() method removes the first occurrence of a specified element from a list.
2. What happens if the specified element is not found in the Python list?
The remove() method raises a ValueError if the specified element is not found.
3. Does the Python list remove() method modify the original list?
Yes, the remove() method modifies the original list by removing the specified element.
4. What types of elements can be removed using the Python list remove() method?
Any type of element (e.g., string, number, list, tuple) can be removed using the remove() method.
5. Can the Python list remove() method remove multiple occurrences of an element in the list?
No, the remove() method only removes the first occurrence of the specified element.
6. Does the Python list remove() method return any value?
No, the remove() method does not return any value. It only updates the list.
7. Is the Python list remove() method case-sensitive when dealing with strings?
Yes, the remove() method is case-sensitive when removing string elements.
8. Can the Python list remove() method be used to remove elements from nested lists?
Yes, the remove() method can remove elements from nested lists if the exact element is specified.
9. How can one handle errors if the element to be removed is not in the Python list?
To handle errors, use a try-except block to catch the ValueError exception.
10. Is the Python list remove() method exclusive to lists?
Yes, the remove() method is specific to lists in Python. Other data structures may have their own methods for removing elements.
Python Code Editor:
Previous: Python List pop() Method.
Next: Python List reverse() Method.
Test your Python skills with w3resource's quiz
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics