Python List reverse() Method
reverse() Method
The reverse() method is used to reverse the elements of a given list.
The reverse() method is a straightforward and efficient way to reverse the order of elements in a list. It is useful in various scenarios where the order of elements needs to be inverted. This method is easy to use, and understanding its behavior is essential for effective list manipulation in Python.
Visual Explanation:
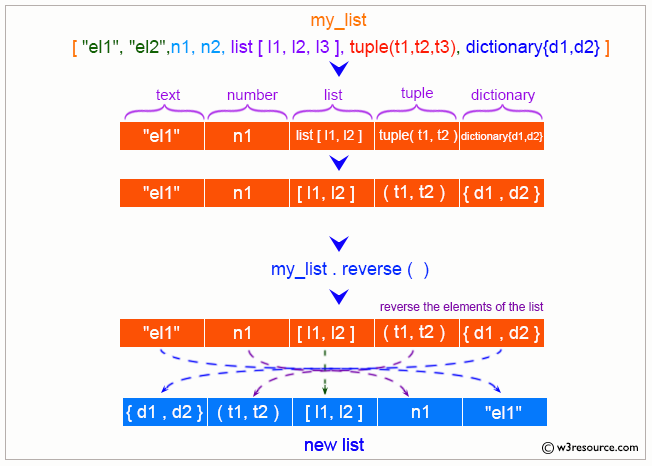
Syntax:
list.reverse()
Parameter: None. The method does not take any parameters.
Return Value: The reverse() method does not return any value. It modifies the original list.
Example 1: Reversing a List
# colors list
colors = ['Red', 'Green', 'Orange', 'Pink']
print("Original List:")
print(colors)
# Reverse the list
colors.reverse()
print("Updated List:")
print(colors)
Output:
Original List: ['Red', 'Green', 'Orange', 'Pink'] Updated List: ['Pink', 'Orange', 'Green', 'Red']
Example 2: Reversing a List of Numbers
# numbers list
numbers = [1, 2, 3, 4, 5]
print("Original List:")
print(numbers)
# Reverse the list
numbers.reverse()
print("Updated List:")
print(numbers)
Output:
Original List: [1, 2, 3, 4, 5] Updated List: [5, 4, 3, 2, 1]
Example 3: Reversing a List of Mixed Data Types
# mixed list
mixed_list = [1, 'a', 3.5, 'Hello']
print("Original List:")
print(mixed_list)
# Reverse the list
mixed_list.reverse()
print("Updated List:")
print(mixed_list)
Output:
Original List: [1, 'a', 3.5, 'Hello'] Updated List: ['Hello', 3.5, 'a', 1]
Example 4: Reversing a List of Lists
# list of lists
list_of_lists = [[1, 2], [3, 4], [5, 6]]
print("Original List:")
print(list_of_lists)
# Reverse the list
list_of_lists.reverse()
print("Updated List:")
print(list_of_lists)
Output:
Original List: [[1, 2], [3, 4], [5, 6]] Updated List: [[5, 6], [3, 4], [1, 2]]
Python list reverse() Method FAQ
1. What does the Python list reverse() method do?
The reverse() method reverses the order of elements in a list in place.
2. Does the Python list reverse() method modify the original list?
Yes, the reverse() method modifies the original list by reversing the order of its elements.
3. Does the Python list reverse() method return any value?
No, the reverse() method does not return any value. It updates the list in place.
4. Can the Python list reverse() method be used on lists with mixed data types?
Yes, the reverse() method can be used on lists containing elements of mixed data types.
5. Are there any parameters required for the Python list reverse() method?
No, the reverse() method does not take any parameters.
6. Can the Python list reverse() method be used on a list of lists?
Yes, the reverse() method can reverse a list of lists, treating each sublist as an individual element.
7. How does the Python list reverse() method handle empty lists?
The reverse() method can be used on empty lists, and it will simply leave the list unchanged.
8. Is the Python list reverse() method available for other data structures like tuples or sets?
No, the reverse() method is specific to lists. Tuples and sets do not have a reverse() method.
9. Is there an alternative way to reverse a list in Python list method?
Yes, a list can be reversed using slicing with the [::-1] syntax or the reversed() function for creating an iterator.
10. What is the time complexity of the Python reverse() method?
The reverse() method has a time complexity of O(n), where n is the number of elements in the list.
Python Code Editor:
Previous: Python List remove() Method.
Next: Python List sort() Method
Test your Python skills with w3resource's quiz
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics